Query Answering (Q&A) is without doubt one of the signature sensible functions of pure language processing. In a earlier put up, you could have seen easy methods to use DistilBERT for query answering by constructing a pipeline utilizing the transformers library. On this put up, you’ll deep dive into the technical particulars to see how one can manipulate the query on your personal function. Particularly, you’ll be taught:
- How you can use the mannequin to reply questions from a context
- How you can interpret the mannequin’s output
- How you can construct your personal question-answering algorithm by leveraging the mannequin’s output
Let’s get began.
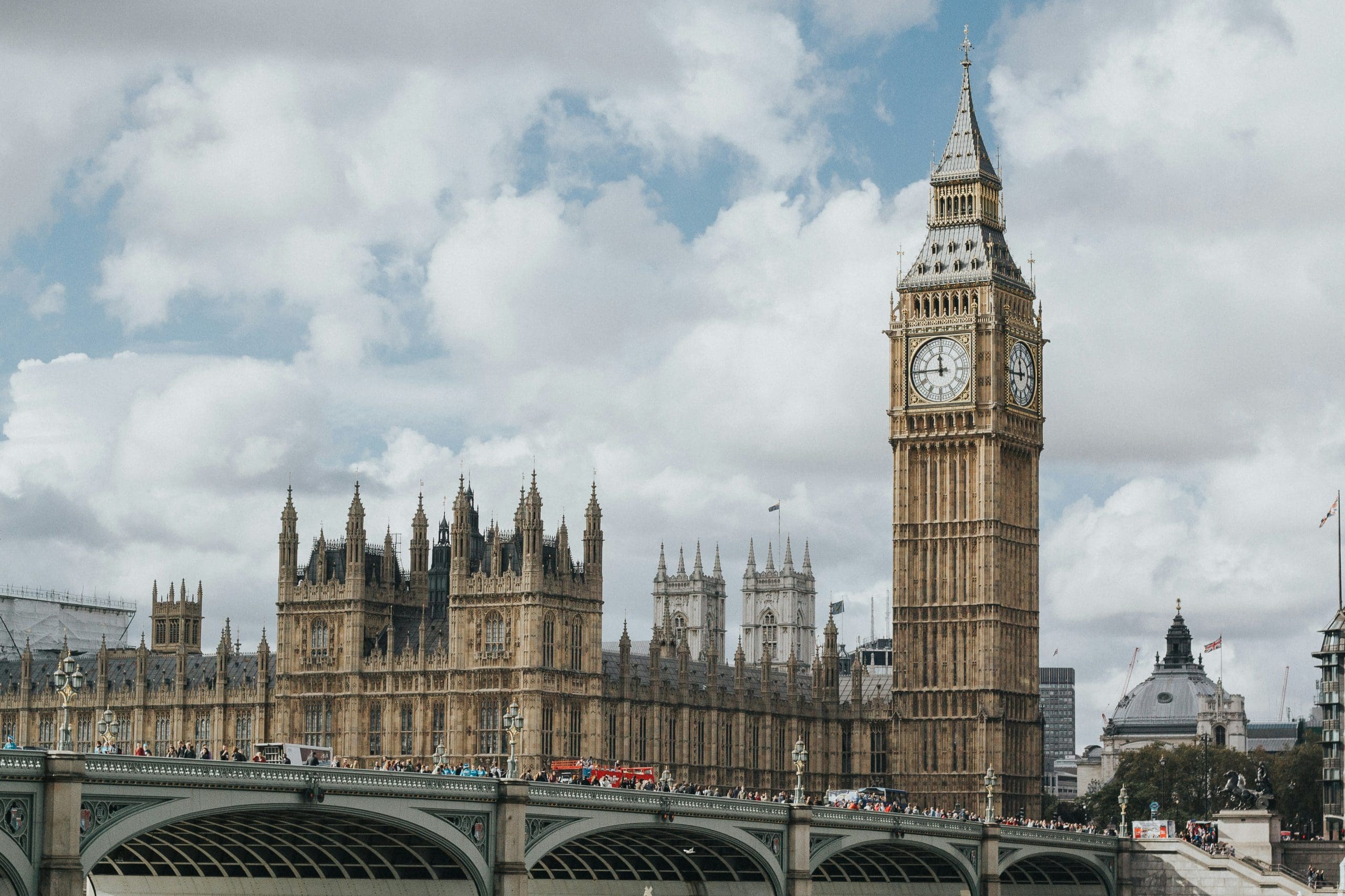
Superior Q&A Options with DistilBERT
Photograph by Marcin Nowak. Some rights reserved.
Overview
This put up is split into three elements; they’re:
- Utilizing DistilBERT Mannequin for Query Answering
- Evaluating the Reply
- Different Methods for Enhancing the Q&A Functionality
Utilizing DistilBERT Mannequin for Query Answering
BERT (Bidirectional Encoder Representations from Transformers) was skilled to be a general-purpose language mannequin that may perceive textual content. DistilBERT is a distilled model, which means it’s architecturally comparable however smaller than BERT. It’s 40% smaller in dimension and runs 60% sooner, whereas its language understanding capabilities are 97% of these of BERT. Due to this fact, it’s a good mannequin for manufacturing use to get larger throughput.
There’s a pre-trained mannequin of DistilBERT within the Hugging Face mannequin hub. It must be used with a selected tokenizer. Within the transformers library, the DistilBERT tokenizer and the Q&A mannequin are respectively DistilBertTokenizer
and DistilBertForQuestionAnswering
. You’ll be able to load the pre-trained mannequin and use it by following the code beneath:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
from transformers import DistilBertTokenizer, DistilBertForQuestionAnswering import torch
# Load pre-trained mannequin and tokenizer model_name = ‘distilbert-base-uncased-distilled-squad’ tokenizer = DistilBertTokenizer.from_pretrained(model_name) mannequin = DistilBertForQuestionAnswering.from_pretrained(model_name)
# Outline a context and a query query = “What’s machine studying?” context = “”“Machine studying is a area of inquiry dedicated to understanding and constructing strategies that “be taught‘, that’s, strategies that leverage knowledge to enhance efficiency on some set of duties. It’s seen as part of synthetic intelligence. Machine studying algorithms construct a mannequin based mostly on pattern knowledge, referred to as coaching knowledge, with the intention to make predictions or selections with out being explicitly programmed to take action. Machine studying algorithms are utilized in all kinds of functions, akin to in drugs, e mail filtering, speech recognition, and pc imaginative and prescient, the place it’s tough or unfeasible to develop typical algorithms to carry out the wanted duties.”””
# Tokenize the enter and run the mannequin inputs = tokenizer(query, context, return_tensors=”pt“) with torch.no_grad(): outputs = mannequin(**inputs)
# Course of the reply answer_start = torch.argmax(outputs.start_logits) answer_end = torch.argmax(outputs.end_logits) answer_tokens = inputs.input_ids[0, answer_start: answer_end + 1] reply = tokenizer.decode(answer_tokens)
print(f“Query: {query}”) print(f“Reply: {reply}”) |
You created the tokenizer and the mannequin utilizing the from_pretrained()
technique. This may obtain the mannequin from the mannequin hub and create the objects. You outlined the query and the context as Python strings. However for the reason that mannequin as a neural community accepts numerical tensors, it is advisable to use a tokenizer to transform the strings into integer “tokens”, which may be understood by the mannequin.
You move on each the query and the context to the tokenizer. This utilization is barely one of many some ways the tokenizer can be utilized however it’s what the Q&A mannequin expects. The tokenizer will perceive the inputs as:
[CLS] query [SEP] context [SEP] |
and [CLS]
and [SEP]
are particular tokens which are used to point the start and finish of the subsequence.
This output is then handed on to the mannequin, which returns an output object with attributes start_logits
and end_logits
. These are logits (log-probabilities) for the place the reply is positioned within the context. Therefore we will extract the subsequence from the sequence of enter tokens, convert it again to textual content, and report that as the reply.
Evaluating the Reply
Recall that the mannequin produces logits (i.e., log chances) for the beginning and finish positions of the reply within the context. The way in which that we extract the reply within the instance above is just to take probably the most possible begin and finish positions utilizing the argmax()
operate. Not that you shouldn’t interpret the floating level values produced by the mannequin as chances. As a way to get the likelihood, it is advisable to convert the logits utilizing the softmax operate.
A really perfect mannequin ought to produce the likelihood as binary, i.e., just one ingredient is likelihood 1, and the remainder are all likelihood 0. In observe, this isn’t the case. As an alternative, the mannequin will produce a likelihood distribution with drastic distinction between one ingredient and the remainder if the mannequin is assured concerning the reply, however virtually a uniform distribution if the mannequin just isn’t assured.
Due to this fact, we will additional interpret the logit output as the arrogance rating of the reply as produced by the mannequin. Under is an instance of why that is helpful:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
from transformers import DistilBertTokenizer, DistilBertForQuestionAnswering import torch import numpy as np
# Load pre-trained mannequin and tokenizer model_name = ‘distilbert-base-uncased-distilled-squad’ tokenizer = DistilBertTokenizer.from_pretrained(model_name) mannequin = DistilBertForQuestionAnswering.from_pretrained(model_name)
# Outline a number of contexts query = “What’s deep studying?” contexts = [ “”“Machine learning is a field of inquiry devoted to understanding and building methods that “learn“, that is, methods that leverage data to improve performance on some set of tasks. It is seen as a part of artificial intelligence.”“”,
“”“Deep learning is a subset of machine learning where artificial neural networks, algorithms inspired by the human brain, learn from large amounts of data. Deep learning is behind many recent advances in AI, including computer vision and speech recognition.”“”,
“”“Natural Language Processing (NLP) is a field of AI that gives machines the ability to read, understand, and derive meaning from human languages. It’s used in applications like chatbots, translation services, and sentiment analysis.”“” ]
# Operate to get reply from a single context def get_answer(query, context): inputs = tokenizer(query, context, return_tensors=“pt”)
with torch.no_grad(): outputs = mannequin(**inputs)
# Get the most certainly reply span answer_start = torch.argmax(outputs.start_logits) answer_end = torch.argmax(outputs.end_logits)
# Calculate the arrogance rating (simplified) confidence = float(outputs.start_logits[0, answer_start] + outputs.end_logits[0, answer_end])
# Extract the reply answer_tokens = inputs.input_ids[0, answer_start: answer_end + 1] reply = tokenizer.decode(answer_tokens)
return reply, confidence
# Get solutions from all contexts answers_with_scores = [get_answer(question, context) for context in contexts]
# Discover the reply with the best confidence rating best_answer_idx = np.argmax([score for _, score in answers_with_scores]) best_answer, best_score = answers_with_scores[best_answer_idx]
print(f“Query: {query}”) print(f“Finest Reply: {best_answer}”) print(f“From Context: {contexts[best_answer_idx][:100]}…”) print(f“Confidence Rating: {best_score}”) |
Within the instance above, as a substitute of 1 query and one context, we offered a listing of contexts and a single query. Every context is used to get a solution and the arrogance rating. That is carried out by the operate get_answer()
. The rating is an easy sum of the mannequin’s predicted worth for the beginning and finish positions, assuming that the mannequin will produce a better worth for a extra assured reply. Lastly, you discover the one with the best confidence rating and report that as the reply.
This strategy permits us to seek for solutions throughout a number of paperwork and return probably the most assured reply. Nevertheless, it’s value noting that this can be a simplified strategy. In a manufacturing system, you may wish to use extra subtle strategies for rating solutions, akin to contemplating the size of the reply and the place within the doc or utilizing a separate rating mannequin.
Different Methods for Enhancing the Q&A Functionality
You’ll be able to simply prolong the code above for a extra subtle Q&A system, akin to one which helps caching the end result or processing a number of questions in a batch.
One limitation of the mannequin used within the instance above is that you just can not feed a really lengthy context to the mannequin. This mannequin has a most sequence size of 512 tokens. In case your context is longer than this, you’ll want to separate it into smaller chunks.
You’ll be able to create chunks naively by splitting the context at each 512 tokens, however you threat breaking the reply in the course of a sentence. One other strategy is to make use of a sliding window. Under is an implementation:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 |
from transformers import DistilBertTokenizer, DistilBertForQuestionAnswering import torch import numpy as np
# Load pre-trained mannequin and tokenizer model_name = “distilbert-base-uncased-distilled-squad” tokenizer = DistilBertTokenizer.from_pretrained(model_name) mannequin = DistilBertForQuestionAnswering.from_pretrained(model_name)
# Outline an extended context query = “What’s the capital of France?” long_context = “”“Paris is the capital and most populous metropolis of France, with an estimated inhabitants of two,175,601 residents as of 2018, in an space of greater than 105 sq. kilometres. The Metropolis of Paris is the centre and seat of presidency of the area and province of Île-de-France, or Paris Area, which has an estimated inhabitants of 12,174,880, or about 18 p.c of the inhabitants of France as of 2017.”“”
def get_answer_sliding_window(query, context, total_len=512, stride=128): “”“Operate to get reply utilizing sliding window”“” # Tokenize the query and context question_tokens = tokenizer.tokenize(query) context_tokens = tokenizer.tokenize(context)
# If the context is brief sufficient, course of it instantly if len(question_tokens) + len(context_tokens) + 3 total_len: # +3 for [CLS], [SEP], [SEP] best_answer, best_score = get_answer(query, context) return best_answer, best_score, context
# In any other case, use sliding window max_question_len = 64 # Restrict query size to make sure we’ve got sufficient area for context if len(question_tokens) > max_question_len: question_tokens = question_tokens[:max_question_len]
# Calculate what number of tokens we will allocate to the context max_len = total_len – len(question_tokens) – 3 # -3 for [CLS], [SEP], [SEP] home windows = [] for i in vary(0, len(context_tokens), stride): home windows.append(tokenizer.convert_tokens_to_string(context_tokens[i:i+max_len])) if i + max_len >= len(context_tokens): break # Final window
# Get solutions from all home windows answers_with_scores = [get_answer(question, window) for window in windows]
# Discover the reply with the best confidence rating best_answer_idx = np.argmax([score for _, score in answers_with_scores]) best_answer, best_score = answers_with_scores[best_answer_idx] return best_answer, best_score, home windows[best_answer_idx]
def get_answer(query, context): “”“Operate to get reply from a single context”“” inputs = tokenizer(query, context, return_tensors=“pt”)
with torch.no_grad(): outputs = mannequin(**inputs)
answer_start = torch.argmax(outputs.start_logits) answer_end = torch.argmax(outputs.end_logits)
confidence = float(outputs.start_logits[0, answer_start] + outputs.end_logits[0, answer_end]) answer_tokens = inputs.input_ids[0, answer_start: answer_end + 1] reply = tokenizer.decode(answer_tokens) return reply, confidence
# Get reply utilizing sliding window best_answer, best_score, best_window = get_answer_sliding_window(query, long_context)
print(f“Query: {query}”) print(f“Finest Reply: {best_answer}”) print(f“From Window: {best_window[:100]}…”) print(f“Confidence Rating: {best_score}”) |
This code implements the operate get_answer_sliding_window()
that may break up the context into shorter items whether it is too lengthy. Every break up to keep up the utmost complete variety of tokens of the query and the context.
The break up is finished by a sliding window, and every break up will transfer the window the dimensions of stride, which is defaulted to 128. In different phrases, every subsequent window will discard 128 tokens from the left and add 128 tokens from the precise. Given the full size of 512, there must be important overlap between the home windows in order that the reply shouldn’t be fragmented in a minimum of one of many home windows.
Reply if discovered utilizing the mannequin with context from every window. One of the best reply is reported based mostly on the arrogance rating. This manner, the context may be of arbitrary size, due to the supply of the tokenizer as an impartial object that you need to use to encode and decode textual content.
One other method to enhance the Q&A functionality is to make use of not one however a number of fashions. That is the thought of ensemble strategies. One easy strategy is to run the query twice, every from a unique mannequin, and choose the reply with the best rating. An instance is proven beneath, which makes use of the unique BERT mannequin because the second mannequin:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
from transformers import DistilBertTokenizer, DistilBertForQuestionAnswering, BertTokenizer, BertForQuestionAnswering import torch
# Load DistilBERT mannequin and tokenizer distilbert_model_name = “distilbert-base-uncased-distilled-squad” distilbert_tokenizer = DistilBertTokenizer.from_pretrained(distilbert_model_name) distilbert_model = DistilBertForQuestionAnswering.from_pretrained(distilbert_model_name)
# Load BERT mannequin and tokenizer bert_model_name = “bert-large-uncased-whole-word-masking-finetuned-squad” bert_tokenizer = BertTokenizer.from_pretrained(bert_model_name) bert_model = BertForQuestionAnswering.from_pretrained(bert_model_name)
# Outline a context and a query query = “What’s the capital of France?” context = “”“Paris is the capital and most populous metropolis of France, with an estimated inhabitants of two,175,601 residents as of 2018, in an space of greater than 105 sq. kilometres. The Metropolis of Paris is the centre and seat of presidency of the area and province of Île-de-France, or Paris Area, which has an estimated inhabitants of 12,174,880, or about 18 p.c of the inhabitants of France as of 2017.”“”
# Operate to get reply from DistilBERT def get_distilbert_answer(query, context): inputs = distilbert_tokenizer(query, context, return_tensors=“pt”)
with torch.no_grad(): outputs = distilbert_model(**inputs)
begin = torch.argmax(outputs.start_logits) finish = torch.argmax(outputs.end_logits)
confidence = float(outputs.start_logits[0, start] + outputs.end_logits[0, end]) answer_tokens = inputs.input_ids[0, start:end+1] reply = distilbert_tokenizer.decode(answer_tokens)
return reply, confidence
# Operate to get reply from BERT def get_bert_answer(query, context): inputs = bert_tokenizer(query, context, return_tensors=“pt”)
with torch.no_grad(): outputs = bert_model(**inputs)
begin = torch.argmax(outputs.start_logits) finish = torch.argmax(outputs.end_logits)
confidence = float(outputs.start_logits[0, start] + outputs.end_logits[0, end])
answer_tokens = inputs.input_ids[0, start:end+1] reply = bert_tokenizer.decode(answer_tokens)
return reply, confidence
# Get solutions from each fashions distilbert_answer, distilbert_confidence = get_distilbert_answer(query, context) bert_answer, bert_confidence = get_bert_answer(query, context)
# Easy ensemble: select the reply with the best confidence if distilbert_confidence > bert_confidence: final_answer = distilbert_answer model_used = “DistilBERT” confidence = distilbert_confidence else: final_answer = bert_answer model_used = “BERT” confidence = bert_confidence
print(f“Query: {query}”) print(f“Ultimate Reply: {final_answer}”) print(f“Mannequin Used: {model_used}”) print(f“Confidence Rating: {confidence}”) |
This code instantiated two tokenizers and two fashions; one is DistilBERT, and the opposite is BERT. The capabilities get_distilbert_answer()
and get_bert_answer()
are used to get the reply from the respective mannequin. Each capabilities are invoked for the offered query and context. The ultimate reply is the one with the best confidence rating.
Ensemble strategies can enhance accuracy by leveraging the strengths of various fashions and mitigating their particular person weaknesses. The above is only one strategy to mix fashions. There are different approaches to make use of with ensemble strategies. For instance, with extra fashions, you need to use voting to decide on probably the most incessantly occurring reply. It’s also possible to assign weights to every mannequin and take the weighted common of their output, from which the reply is derived. A extra difficult strategy is stacking, the place you prepare a meta-model to mix the predictions of the bottom fashions. This generalized the weighted common strategy however elevated the computational complexity.
Additional Readings
Under are some additional readings that you could be discover helpful:
Abstract
On this put up, you could have explored easy methods to use DistilBERT for superior question-answering duties. Particularly, you could have realized:
- How you can use DistilBERT’s tokenizer and the Q&A mannequin instantly
- How you can interpret the Q&A mannequin’s output and extract the reply
- How one can make use of the mannequin’s uncooked output to construct a extra subtle Q&A system
Source link