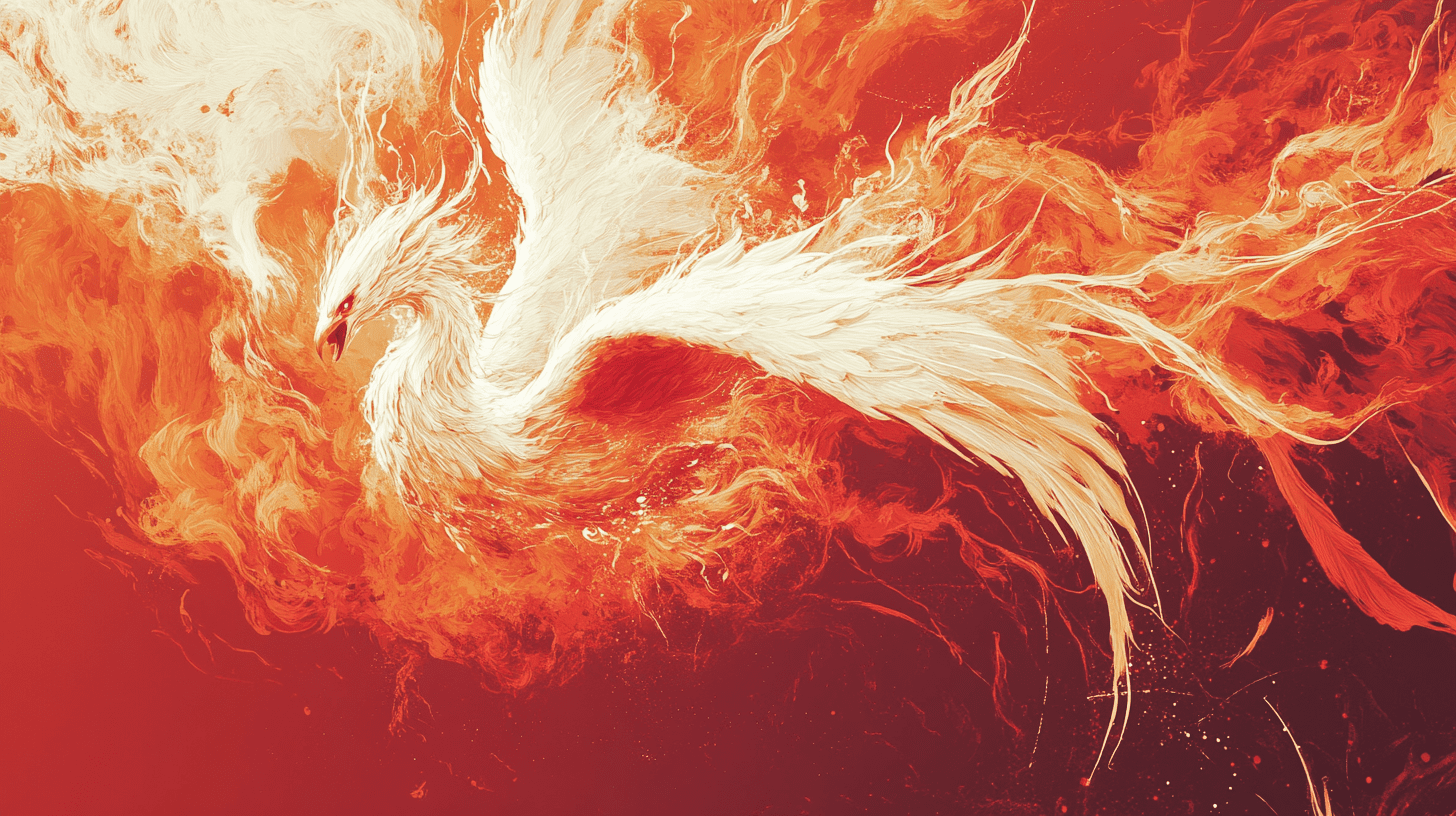
Creating Customized Layers and Loss Capabilities in PyTorch
Picture by Editor | Midjourney
Creating customized layers and loss capabilities in PyTorch is a elementary ability for constructing versatile and optimized deep studying fashions. Whereas PyTorch supplies a sturdy library of predefined layers and loss capabilities, there are eventualities the place tailoring these parts to your particular drawback can result in higher efficiency and explainability.
With this in thoughts, we’ll discover the necessities of making and integrating custom layers and loss functions in PyTorch, illustrated with code snippets and sensible insights.
Understanding the Want for Customized Parts
PyTorch’s predefined modules and capabilities are extremely versatile, however real-world issues usually demand improvements past customary instruments. Customized layers and loss capabilities can:
- Deal with domain-specific necessities: For instance, duties involving irregular information buildings or specialised metrics might profit from distinctive transformations or analysis strategies
- Improve mannequin efficiency: Tailoring layers or losses to your drawback can result in higher convergence, larger accuracy, or decrease computational prices
- Incorporate area data: By embedding domain-specific insights straight into the mannequin, you possibly can enhance interpretability and alignment with real-world eventualities
Whereas fundamental use instances would possibly see the introduction of customized layers and losses as overkill, it’s tailored for industries like healthcare and logistics. Likewise, finance is one other potential discipline the place we would see PyTorch use taking off. Even easy duties like extracting information from invoices require dealing with irregular information, with pc imaginative and prescient fashions already making strides for functions like this.
Customized Layers in PyTorch
Customized layers allow you to outline particular transformations or operations that are not available in PyTorch’s standard library. This may be helpful in duties involving distinctive information processing necessities, comparable to modeling irregular patterns or making use of domain-specific logic.
Step 1: Outline the Layer Class
In PyTorch, all customized layers are applied by subclassing torch.nn.Module
and defining two key strategies:
__init__
: Initialize the parameters or sub-modules utilized by the layerahead
: Outline the ahead cross logic
Right here’s an instance of a customized linear layer:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
import torch import torch.nn as nn
class CustomLinear(nn.Module): def __init__(self, input_dim, output_dim): tremendous(CustomLinear, self).__init__() self.weight = nn.Parameter(torch.randn(output_dim, input_dim)) self.bias = nn.Parameter(torch.randn(output_dim)) def ahead(self, x): return torch.matmul(x, self.weight.T) + self.bias
# Instance utilization x = torch.randn(10, 5) # Batch of 10 samples, every with 5 options custom_layer = CustomLinear(input_dim=5, output_dim=3) output = custom_layer(x)
print(output.form) # Output >> torch.Dimension([10, 3]) |
This layer performs a linear transformation however is absolutely customizable, permitting for additional diversifications if wanted.
Step 2: Add Superior Performance
Customized layers may also embody non-linear transformations or particular operations. As an example, a customized ReLU layer with a configurable threshold might appear to be this:
class ThresholdReLU(nn.Module): def __init__(self, threshold=0.0): tremendous(ThresholdReLU, self).__init__() self.threshold = threshold def ahead(self, x): return torch.the place(x > self.threshold, x, torch.zeros_like(x))
# Instance utilization relu_layer = ThresholdReLU(threshold=0.5) x = torch.tensor([[–1.0, 0.3], [0.6, 1.2]]) output = relu_layer(x)
print(output) # Output >> tensor([[0.0000, 0.0000], [0.6000, 1.2000]]) |
This highlights the pliability PyTorch supplies for implementing domain-specific operations.
Step 3: Combine Customized Layers
Customized layers will be seamlessly built-in into fashions by together with them as sub-modules in bigger architectures. As an example:
class CustomModel(nn.Module): def __init__(self): tremendous(CustomModel, self).__init__() self.layer1 = nn.Linear(5, 10) self.custom_layer = CustomLinear(10, 3) self.output_layer = nn.Linear(3, 1) def ahead(self, x): x = torch.relu(self.layer1(x)) x = self.custom_layer(x) return self.output_layer(x)
mannequin = CustomModel() |
This modular strategy ensures the maintainability and reusability of your customized parts.
Customized Loss Capabilities
A custom loss function is crucial when predefined choices like imply squared error or cross-entropy don’t align with the precise necessities of your mannequin. As well as, we’ll check out duties requiring non-standard distance metrics or domain-specific analysis standards.
Step 1: Outline the Loss Class
Much like customized layers, customized loss capabilities are applied by subclassing torch.nn.Module
. The secret is to outline the ahead technique that computes the loss primarily based on inputs.
Right here’s an instance of a customized loss operate that penalizes massive outputs:
class CustomLoss(nn.Module): def __init__(self): tremendous(CustomLoss, self).__init__() def ahead(self, predictions, targets): mse_loss = torch.imply((predictions – targets) ** 2) penalty = torch.imply(predictions ** 2) return mse_loss + 0.1 * penalty
# Instance utilization predictions = torch.randn(10, 1) targets = torch.randn(10, 1) loss_fn = CustomLoss() loss = loss_fn(predictions, targets) print(loss) |
The penalty time period encourages smaller predictions, a helpful characteristic in sure regression issues.
Step 2: Prolong Performance
You possibly can design loss capabilities for extra advanced metrics. For instance, think about a customized loss that mixes MAE and cosine similarity:
class CombinedLoss(nn.Module): def __init__(self): tremendous(CombinedLoss, self).__init__() def ahead(self, predictions, targets): mae_loss = torch.imply(torch.abs(predictions – targets)) cosine_loss = 1 – torch.nn.practical.cosine_similarity(predictions, targets, dim=0).imply() return mae_loss + cosine_loss
# Instance utilization loss_fn = CombinedLoss() loss = loss_fn(predictions, targets) print(loss) |
This flexibility permits the mixing of a number of metrics for duties requiring nuanced analysis standards.
Combining Customized Layers and Loss
Lastly, let’s observe an instance the place we combine a customized layer and loss operate right into a easy mannequin:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
class ExampleModel(nn.Module): def __init__(self): tremendous(ExampleModel, self).__init__() self.custom_layer = CustomLinear(5, 3) self.output_layer = nn.Linear(3, 1) def ahead(self, x): x = torch.relu(self.custom_layer(x)) return self.output_layer(x) # Information inputs = torch.randn(100, 5) targets = torch.randn(100, 1)
# Mannequin, Loss, Optimizer mannequin = ExampleModel() loss_fn = CustomLoss() optimizer = torch.optim.Adam(mannequin.parameters(), lr=0.01)
# Coaching Loop for epoch in vary(50): optimizer.zero_grad() predictions = mannequin(inputs) loss = loss_fn(predictions, targets) loss.backward() optimizer.step() if epoch % 10 == 0: print(f“Epoch {epoch}, Loss: {loss.merchandise()}”) |
Conclusion
Creating customized layers and loss capabilities in PyTorch empowers you to design extremely tailor-made and efficient fashions. This functionality lets you tackle distinctive challenges and unlock higher efficiency in your deep studying workflows.
You should definitely think about these debugging and optimization solutions when working by yourself customized layers and loss capabilities.
- Validate parts independently: Use synthetic data to confirm the performance of your customized layers and loss capabilities
- Leverage PyTorch instruments: Use
torch.autograd.gradcheck
to confirm gradients andtorch.profiler
for efficiency profiling - Optimize implementations: Refactor computationally intensive operations utilizing vectorized implementations for higher efficiency
Combining flexibility with PyTorch’s wealthy ecosystem ensures that your fashions stay scalable, interpretable, and aligned with the precise calls for of your utility.
Source link