Textual content embeddings have revolutionized pure language processing by offering dense vector representations that seize semantic that means. Within the previous tutorial, you discovered the way to generate these embeddings utilizing transformer fashions. On this put up, you’ll be taught the superior functions of textual content embeddings that transcend fundamental duties like semantic search and doc clustering.
Particularly, you’ll be taught:
- Find out how to construct suggestion methods utilizing textual content embeddings
- Find out how to implement cross-lingual functions with multilingual embeddings
- Find out how to create textual content classification methods with embedding-based options
- Find out how to develop zero-shot studying functions
- Find out how to visualize and analyze textual content embeddings
Let’s get began.
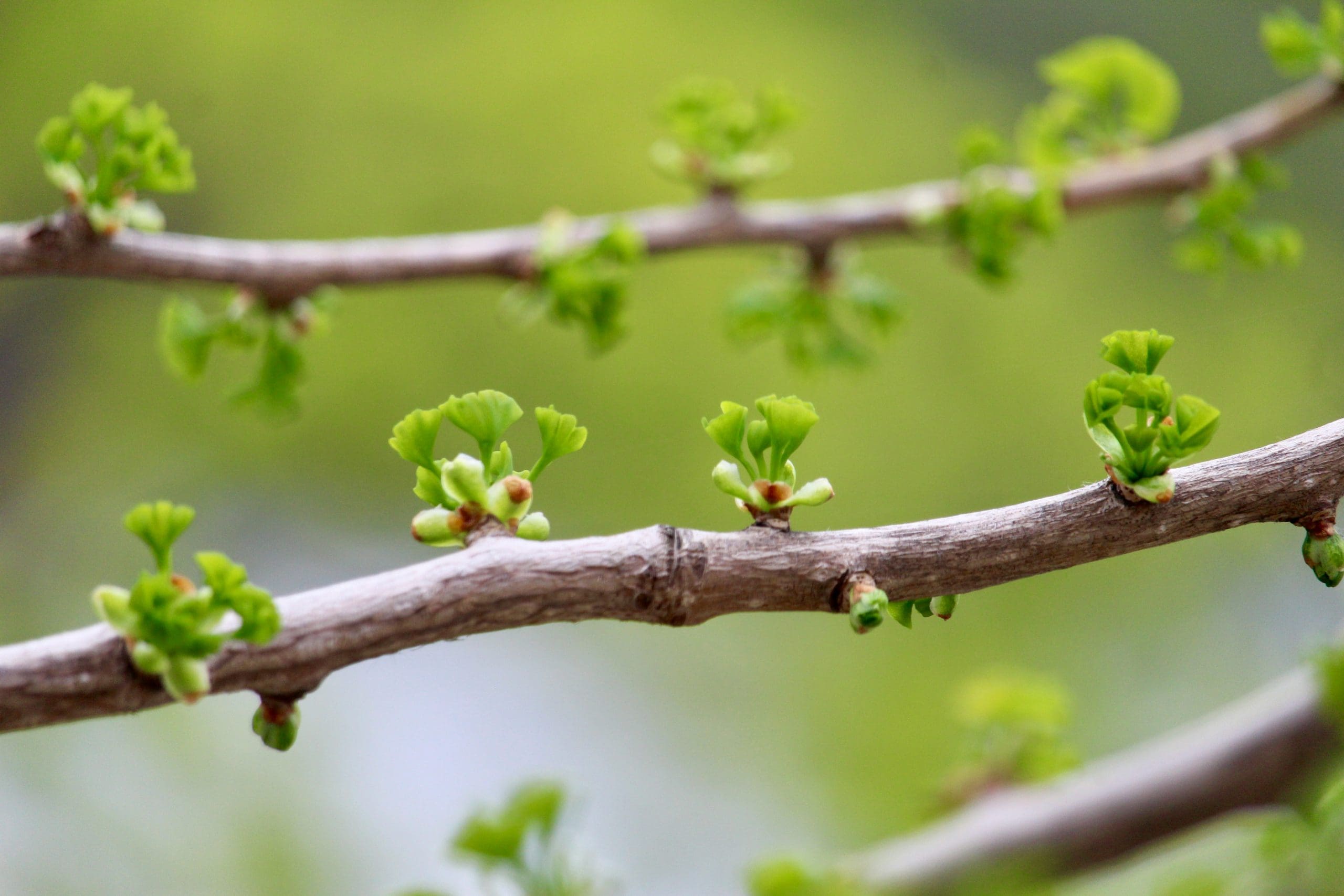
Instance Functions of Textual content Embedding
Picture by Christina Winter. Some rights reserved.
Overview
This put up is split into 5 elements; they’re:
- Suggestion Techniques
- Cross-Lingual Functions
- Textual content Classification
- Zero-Shot Classification
- Visualizing Textual content Embeddings
Suggestion Techniques
A easy suggestion system might be created by discovering just a few of probably the most related gadgets to the goal merchandise. Within the instance of pure language processing, yow will discover some related articles as “you may additionally like” whereas the consumer is studying an article.
There are a lot of methods to implement this. However the easiest method is to verify how related are the 2 articles. You possibly can simply convert all of the articles right into a context embedding. The 2 articles with the best similarity within the context embedding are related in content material. It might not be what you count on for a suggestion, however it’s generally helpful and it’s a good place to begin.
Let’s implement this as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
import numpy as np from sentence_transformers import SentenceTransformer from sklearn.metrics.pairwise import cosine_similarity
# Outline a corpus of articles (title and content material) articles = [ { “title”: “Understanding Deep Learning”, “content”: (“Deep learning is a subset of machine learning where artificial neural networks, “ “algorithms inspired by the human brain, learn from large amounts of data.”) }, { “title”: “Introduction to Natural Language Processing”, “content”: (“Natural Language Processing (NLP) is a field of AI that gives machines the “ “ability to read, understand, and derive meaning from human languages.”) }, { “title”: “The Future of Computer Vision”, “content”: (“Computer vision is an interdisciplinary field that deals with how computers can “ “gain high-level understanding from digital images or videos.”) }, { “title”: “Reinforcement Learning Explained”, “content”: (“Reinforcement learning is an area of machine learning concerned with how “ “software agents ought to take actions in an environment so as to maximize some “ “notion of cumulative reward.”) }, { “title”: “Neural Networks and Their Applications”, “content”: (“Neural networks are a set of algorithms, modeled loosely after the human brain, “ “that are designed to recognize patterns in data.”) } ]
mannequin = SentenceTransformer(“all-MiniLM-L6-v2”)
def create_article_embeddings(articles, mannequin): “”“create embeddings for articles”“” texts = [f“{article[“title“]}. {article[“content“]}” for article in articles] embeddings = mannequin.encode(texts) return embeddings
def get_recommendations(article_id, articles, embeddings, top_n=2): “”“get suggestions for a given article ID primarily based on cosine similarity”“” similarities = cosine_similarity([embeddings[article_id]], embeddings)[0] similar_indices = np.argsort(similarities)[::–1][1:top_n+1] return [articles[idx] for idx in similar_indices]
# Create embeddings for all articles, and get suggestion for first article embeddings = create_article_embeddings(articles, mannequin) suggestions = get_recommendations(0, articles, embeddings)
# Print the suggestions print(f‘Suggestions for “{articles[0][“title”]}”:’) for i, rec in enumerate(suggestions): print(f“{i+1}. {rec[“title“]}”) |
You arrange a corpus originally of the code as a result of it’s a toy instance. In follow, chances are you’ll wish to retrieve the corpus from a database or from a file system.
On this program, you used the all-MiniLM-L6-v2
mannequin and instantiated it with SentenceTransformer
. This can be a pre-trained mannequin that may encode a textual content right into a context embedding. You are taking all of the articles outlined within the corpus and convert every of them right into a context embedding within the operate create_article_embeddings()
. The output is a vector of vectors, or a matrix. On this specific implementation, there are 5 gadgets within the corpus, and the embedding vector has 384 dimensions. The output embeddings
is a matrix of form (5, 384).
In get_recommendations()
, you calculate the cosine similarity between one embedding and all others. The operate cosine_similarity()
from scikit-learn requires two lists of vectors and returns a matrix saying how related every pair of vectors is. Since you’re evaluating one to all others, the output matrix has solely a single row. Then in np.argsort(similarities)
, you obtained the indices of the similarity rating in ascending order. Since cosine similarity is 1 when the vectors are equivalent and 0 when they’re orthogonal (i.e., completely totally different), you reverse the end result to order the similarity rating in descending order. Then, probably the most related gadgets are these originally of this listing, Besides the primary one, which is the article itself.
When you obtained the indices of probably the most related gadgets, you used a for-loop to print the suggestions.
Whenever you run this code, you’re going to get:
Suggestions for “Understanding Deep Studying”: 1. Neural Networks and Their Functions 2. Reinforcement Studying Defined |
These suggestions can be primarily based on semantic similarity slightly than simply key phrase matching, so you’re going to get articles about neural networks or machine studying even when they don’t comprise the precise phrase “deep studying.” This strategy might be prolonged to extra complicated suggestion methods by incorporating consumer preferences, collaborative filtering, or hybrid approaches.
Cross-Lingual Functions
One of many highly effective options of recent transformer fashions is their capacity to generate embeddings for textual content in a number of languages. This allows cross-lingual functions the place you’ll be able to examine or course of textual content throughout totally different languages.
Let’s implement a easy cross-lingual semantic search system:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
import numpy as np from sentence_transformers import SentenceTransformer from sklearn.metrics.pairwise import cosine_similarity
corpus = [ { “language”: “English”, “text”: (“Machine learning is a field of study that gives computers the ability to learn “ “without being explicitly programmed.”) }, { “language”: “Spanish”, “text”: (“El aprendizaje automático es un campo de estudio que da a las computadoras la “ “capacidad de aprender sin ser programadas explícitamente.”) }, { “language”: “French”, “text”: (“L’apprentissage automatique est un domaine d’étude qui donne aux ordinateurs “ “la capacité d’apprendre sans être explicitement programmés.”) }, { “language”: “German”, “text”: (“Maschinelles Lernen ist ein Studienbereich, der Computern die Fähigkeit gibt, “ “zu lernen, ohne explizit programmiert zu werden.”) }, { “language”: “Italian”, “text”: (“Il machine learning è un campo di studio che conferisce ai computer la capacità “ “di apprendere senza essere esplicitamente programmati.”) }, { “language”: “English”, “text”: (“Natural language processing is a subfield of linguistics, computer science, “ “and artificial intelligence.”) }, { “language”: “English”, “text”: (“Computer vision is an interdisciplinary field that deals with how computers can “ “gain high-level understanding from digital images or videos.”) } ]
mannequin = SentenceTransformer(“paraphrase-multilingual-MiniLM-L12-v2”)
# Generate embeddings for the corpus texts = [doc[“text”] for doc in corpus] embeddings = mannequin.encode(texts)
# Outline a question in English and generate an embedding question = “What’s machine studying?” query_embedding = mannequin.encode(question)
# Type the embeddings of the corpus by descending similarity similarities = cosine_similarity([query_embedding], embeddings)[0] ranked_indices = np.argsort(similarities)[::–1]
# Print ranked outcomes print(f“Question: {question}n”) for i, idx in enumerate(ranked_indices[:3]): # Present prime 3 outcomes print(f“{i+1}. [{corpus[idx][“language“]}] {corpus[idx][“text“]} (Similarity: {similarities[idx]:.4f})”) |
On this instance, we’re utilizing a multilingual Sentence Transformer mannequin (paraphrase-multilingual-MiniLM-L12-v2
) to create embeddings for paperwork in several languages. The corpus accommodates numerous languages and numerous matters. This system above is to implement a question-answering system, however the query could discover the reply in a distinct language.
The instance above is similar to the one within the earlier part. The corpus is first transformed into embeddings. Then the question in its embedding type is in contrast with the corpus by cosine similarity. The highest 3 outcomes are printed. Operating this code provides you with:
Question: What’s machine studying?
1. [Italian] Il machine studying è un campo di studio che conferisce ai pc la capacità di apprendere senza essere esplicitamente programmati. (Similarity: 0.8129) 2. [English] Machine studying is a discipline of research that offers computer systems the power to be taught with out being explicitly programmed. (Similarity: 0.7788) 3. [French] L’apprentissage automatique est un domaine d’étude qui donne aux ordinateurs la capacité d’apprendre sans être explicitement programmés. (Similarity: 0.7470) |
The highest reply is in Italian, whereas the query, “What’s machine studying?” is in English. This works as a result of the embedding vector represents the semantic that means of the textual content, whatever the language. This cross-lingual functionality is especially helpful for functions like multilingual search engines like google.
Textual content Classification
Think about you could have numerous textual content knowledge, and it’s rising every single day. This can be since you are amassing new articles or emails. You wish to classify them into totally different classes. This may be achieved by utilizing textual content embeddings.
This can be a job just like “matter modeling”. Subject modeling is an unsupervised studying job that teams textual content paperwork into totally different matters. It makes use of algorithms like Latent Dirichlet Allocation (LDA) to seek out the signature key phrases for classification. Here’s a supervised strategy: You’ve gotten a predefined set of classes and a few examples (perhaps you do the classification manually). Then you definately add new textual content to the gathering with the classification achieved routinely.
Textual content embeddings may also help by extracting the semantic that means of the textual content into vectors. Then you’ll be able to prepare a machine studying mannequin to categorise the vectors into classes. It really works higher this manner as a result of the vector represents the that means of the textual content slightly than the textual content itself. Therefore, it’s higher than utilizing bag-of-words or TF-IDF options.
There are a lot of methods to implement the machine studying classifier. A easy one is a logistic regression from scikit-learn. Let’s implement this within the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
from sentence_transformers import SentenceTransformer from sklearn.linear_model import LogisticRegression from sklearn.metrics import classification_report from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler
articles = [ # Business articles {“text”: “The stock market reached a new high today, with technology stocks leading the gains.”, “category”: “Business”}, {“text”: “The government announced a new tax policy that will affect small businesses.”, “category”: “Business”}, {“text”: “The central bank has decided to keep interest rates unchanged.”, “category”: “Business”}, {“text”: “Quarterly earnings reports exceeded expectations for most Fortune 500 companies.”, “category”: “Business”}, {“text”: “Inflation rates have decreased for the third consecutive month.”, “category”: “Business”}, {“text”: “The merger between two major corporations has been approved by regulators.”, “category”: “Business”}, {“text”: “Unemployment rates have fallen to a five-year low according to new data.”, “category”: “Business”}, {“text”: “The cryptocurrency market experienced significant volatility this week.”, “category”: “Business”},
# Health articles {“text”: “A new study shows that regular exercise can reduce the risk of heart disease.”, “category”: “Health”}, {“text”: “A clinical trial for a new cancer treatment has shown promising results.”, “category”: “Health”}, {“text”: “A balanced diet and regular sleep are essential for maintaining good health.”, “category”: “Health”}, {“text”: “Medical researchers have identified a new gene linked to Alzheimer’s disease.”, “category”: “Health”}, {“text”: “The WHO has issued new guidelines for managing diabetes in elderly patients.”, “category”: “Health”}, {“text”: “A new technique for early detection of breast cancer has been developed.”, “category”: “Health”}, {“text”: “Studies show that mindfulness meditation can help reduce stress and anxiety.”, “category”: “Health”}, {“text”: “Public health officials warn of a potential flu outbreak this winter season.”, “category”: “Health”},
# Technology articles {“text”: “The latest smartphone from Apple features a better camera and longer battery life.”, “category”: “Technology”}, {“text”: “The new electric car from Tesla has a range of over 400 miles.”, “category”: “Technology”}, {“text”: “The latest update to the operating system includes new security features.”, “category”: “Technology”}, {“text”: “A new artificial intelligence system can detect diseases from medical images.”, “category”: “Technology”}, {“text”: “The tech company unveiled its new virtual reality headset at the annual conference.”, “category”: “Technology”}, {“text”: “Researchers have developed a quantum computer that can solve complex problems.”, “category”: “Technology”}, {“text”: “The new social media platform has gained millions of users in just a few months.”, “category”: “Technology”}, {“text”: “Cybersecurity experts warn of a new type of malware targeting smart home devices.”, “category”: “Technology”},
# Science articles {“text”: “Scientists have discovered a new species of frog in the Amazon rainforest.”, “category”: “Science”}, {“text”: “Astronomers have observed a supernova in a distant galaxy.”, “category”: “Science”}, {“text”: “Researchers have developed a new method for measuring ocean temperatures.”, “category”: “Science”}, {“text”: “A fossil discovery suggests that dinosaurs may have been warm-blooded.”, “category”: “Science”}, {“text”: “Climate scientists report that Arctic ice is melting at an unprecedented rate.”, “category”: “Science”}, {“text”: “Physicists have confirmed the existence of a new subatomic particle.”, “category”: “Science”}, {“text”: “A study of coral reefs shows signs of recovery in protected marine areas.”, “category”: “Science”}, {“text”: “Biologists have sequenced the genome of an endangered species of tiger.”, “category”: “Science”} ]
# Put together knowledge for classification coaching mannequin = SentenceTransformer(“all-MiniLM-L6-v2”) texts = [article[“text”] for article in articles] X = mannequin.encode(texts) y = [article[“category”] for article in articles]
# Normalize options scaler = StandardScaler() X_scaled = scaler.fit_transform(X)
# Cut up knowledge into coaching and testing units with stratification X_train, X_test, y_train, y_test = train_test_split( X_scaled, y, test_size=0.2, random_state=42, stratify=y )
# Prepare a logistic regression classifier with regularization classifier = LogisticRegression(C=1.0, class_weight=“balanced”, max_iter=1000) classifier.match(X_train, y_train)
# Consider the classifier y_pred = classifier.predict(X_test) print(classification_report(y_test, y_pred))
# Classify new articles new_articles = [ “The company reported a 20% increase in quarterly profits.”, “A new vaccine has been approved for use against the flu.”, “The new laptop features a faster processor and more memory.”, “The Mars rover has sent back new images of the planet”s surface.” ] new_embeddings = mannequin.encode(new_articles) new_embeddings_scaled = scaler.rework(new_embeddings) new_predictions = classifier.predict(new_embeddings_scaled) for article, prediction in zip(new_articles, new_predictions): print(f“Article: {article}nPredicted Class: {prediction}n”) |
Whenever you run this, you’re going to get:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
precision recall f1-score help
Enterprise 1.00 1.00 1.00 2 Well being 0.50 1.00 0.67 1 Science 1.00 1.00 1.00 2 Expertise 1.00 0.50 0.67 2
accuracy 0.86 7 macro avg 0.88 0.88 0.83 7 weighted avg 0.93 0.86 0.86 7
Article: The corporate reported a 20% improve in quarterly income. Predicted Class: Enterprise
Article: A brand new vaccine has been accepted to be used towards the flu. Predicted Class: Well being
Article: The brand new laptop computer includes a sooner processor and extra reminiscence. Predicted Class: Expertise
Article: The Mars rover has despatched again new photos of the planet”s floor. Predicted Class: Science |
On this instance, the corpus is annotated with one of many 4 classes: enterprise, well being, expertise, or science. The textual content is transformed into embeddings, which, along with the class label, are used to coach a logistic regression classifier.
The classifier is skilled with 80% of the corpus after which evaluated with the remaining 20%. The outcomes are printed within the type of a classification report. You possibly can see that Enterprise and Science are categorized precisely, however Well being and Expertise are usually not so good. Whenever you end the coaching, you need to use the skilled classifier on the brand new articles. The workflow is similar as in coaching: Encode the textual content into embeddings, then scale the embeddings utilizing the skilled scaler, and eventually, use the skilled classifier to foretell the class.
Word that you need to use different classifiers like random forest or Okay-Nearest Neighbors. You possibly can attempt them and see which one works higher.
Zero-Shot Classification
Within the earlier instance, you skilled a classifier to categorise the textual content into one of many predefined classes. If the class labels are significant textual content, why can’t you utilize the that means of the label for classification? On this method, you’ll be able to merely convert the textual content into embeddings after which examine it with the class labels’ embeddings. The textual content is then tagged with probably the most related class label.
That is the concept of zero-shot studying. It’s not a supervised studying job. Certainly, you by no means prepare a brand new mannequin, however the classification and data retrieval duties can nonetheless be achieved.
Let’s implement a zero-shot textual content classifier utilizing textual content embeddings:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import torch from sentence_transformers import SentenceTransformer, util
texts = [ “The stock market reached a new high today, with technology stocks leading the gains.”, “A new study shows that regular exercise can reduce the risk of heart disease.”, “The latest smartphone from Apple features a better camera and longer battery life.”, “Scientists have discovered a new species of frog in the Amazon rainforest.” ] classes = [“Business”, “Health”, “Technology”, “Science”]
# Load a pre-trained Sentence Transformer mannequin mannequin = SentenceTransformer(“all-MiniLM-L6-v2”) text_embeddings = mannequin.encode(texts, convert_to_tensor=True) category_embeddings = mannequin.encode(classes, convert_to_tensor=True)
# Calculate cosine similarity between texts and classes similarities = util.cos_sim(text_embeddings, category_embeddings)
# Get probably the most related class for every textual content best_categories = torch.argmax(similarities, dim=1) for i, textual content in enumerate(texts): class = classes[best_categories[i]] similarity = similarities[i][best_categories[i]].merchandise() print(f“Textual content: {textual content}”) print(f“Class: {class} (Similarity: {similarity:.4f})n”) |
The output is:
Textual content: The inventory market reached a brand new excessive right now, with expertise shares main the positive aspects. Class: Expertise (Similarity: 0.2624)
Textual content: A brand new research reveals that common train can cut back the chance of coronary heart illness. Class: Well being (Similarity: 0.3297)
Textual content: The newest smartphone from Apple options a greater digital camera and longer battery life. Class: Expertise (Similarity: 0.1623)
Textual content: Scientists have found a brand new species of frog within the Amazon rainforest. Class: Science (Similarity: 0.1940) |
The end result might not be pretty much as good because the earlier instance as a result of the class labels are generally ambiguous, and also you do not need a mannequin skilled for this job. However, it produces significant outcomes.
Zero-shot studying is especially helpful for duties the place labeled coaching knowledge is scarce or unavailable. It may be utilized to a variety of NLP duties, together with classification, entity recognition, and question-answering.
Visualizing Textual content Embeddings
Not a selected utility, however visualizing textual content embeddings can generally present insights into the semantic relationships between texts. Since embeddings sometimes have tons of of dimensions, you want dimensionality discount methods to visualise them in 2D or 3D.
PCA might be the preferred dimensionality discount approach. Nevertheless, for visualization, t-SNE (t-Distributed Stochastic Neighbor Embedding) normally works higher. Let’s implement a visualization of textual content embeddings utilizing t-SNE:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
import matplotlib.pyplot as plt import numpy as np from sentence_transformers import SentenceTransformer from sklearn.manifold import TSNE
texts_with_categories = [ {“text”: “The stock market reached a new high today.”, “category”: “Business”}, {“text”: “Investors are optimistic about the economy.”, “category”: “Business”}, {“text”: “The company reported strong quarterly earnings.”, “category”: “Business”}, {“text”: “The central bank has decided to keep interest rates unchanged.”, “category”: “Business”}, {“text”: “A new study shows that regular exercise can reduce the risk of heart disease.”, “category”: “Health”}, {“text”: “A balanced diet is essential for maintaining good health.”, “category”: “Health”}, {“text”: “The new vaccine has been approved for use against the flu.”, “category”: “Health”}, {“text”: “Sleep is important for physical and mental health.”, “category”: “Health”}, {“text”: “The latest smartphone features a better camera and longer battery life.”, “category”: “Technology”}, {“text”: “The new laptop has a faster processor and more memory.”, “category”: “Technology”}, {“text”: “The software update includes new security features.”, “category”: “Technology”}, {“text”: “5G networks promise faster internet speeds for mobile devices.”, “category”: “Technology”}, {“text”: “Scientists have discovered a new species in the Amazon rainforest.”, “category”: “Science”}, {“text”: “Astronomers have observed a supernova in a distant galaxy.”, “category”: “Science”}, {“text”: “The Mars rover has sent back new images of the planet’s surface.”, “category”: “Science”}, {“text”: “Researchers have developed a new method for measuring ocean temperatures.”, “category”: “Science”} ]
# Extract texts and classes texts = [item[“text”] for merchandise in texts_with_categories] classes = [item[“category”] for merchandise in texts_with_categories]
# Generate embeddings, then cut back dimension with t-SNE mannequin = SentenceTransformer(“all-MiniLM-L6-v2”) embeddings = mannequin.encode(texts)
tsne = TSNE(n_components=2, perplexity=5, random_state=42) reduced_embeddings = tsne.fit_transform(embeddings)
# Outline colours for classes unique_categories = listing(set(classes)) colours = plt.cm.rainbow(np.linspace(0, 1, len(unique_categories))) category_to_color = {class: colour for class, colour in zip(unique_categories, colours)}
# Create a scatter plot plt.determine(figsize=(10, 8)) for i, (x, y) in enumerate(reduced_embeddings): class = classes[i] colour = category_to_color[category] plt.scatter(x, y, colour=colour, alpha=0.7) plt.annotate(texts[i][:20] + “…”, (x, y), fontsize=8)
# Add legend, mark the axes for class, colour in category_to_color.gadgets(): plt.scatter([], [], colour=colour, label=class) plt.legend() plt.xlabel(“t-SNE Dimension 1”) plt.ylabel(“t-SNE Dimension 2”) plt.title(“t-SNE Visualization of Textual content Embeddings”) plt.tight_layout() plt.present() |
You used scikit-learn’s t-SNE implementation. It’s straightforward to make use of as all you could do is to cross the rows of embedding vectors to the tsne.fit_transform()
technique. The output embeddings
is a $N occasions 2$ array (i.e., coordinates in 2D area).
Then, you used a for-loop to plot every reworked embedding into a degree within the scatter plot. Every level is coloured primarily based on the class annotated within the unique textual content. To keep away from cluttering the plot, legends are created afterward in one other for-loop. The plot produced is like the next:
The visualization places texts with related meanings collectively; this implies the labels are helpful to characterize the semantic that means of the texts. You possibly can have a look at the plot and verify if the factors from the identical class are clustered shut sufficient to inform in case your embeddings are good.
Different dimensionality discount methods exist, comparable to PCA (Principal Element Evaluation) or UMAP (Uniform Manifold Approximation and Projection). You possibly can attempt these to see if the visualization nonetheless is sensible.
Additional Readings
Under are some additional readings that you could be discover helpful:
Abstract
On this tutorial, you discovered just a few functions of textual content embeddings. Significantly, you discovered the way to:
- Construct a suggestion system utilizing similarity within the embedding area
- Implement cross-lingual functions with multilingual embeddings
- Prepare a textual content classification system utilizing embedding as options
- Develop a zero-shot textual content labeling utility utilizing similarity metrics in embedding area
- Visualize and analyze textual content embeddings
Textual content embeddings are easy but highly effective instruments for a variety of NLP duties. They allow machines to grasp and course of textual content in a method that captures semantic that means.
Source link