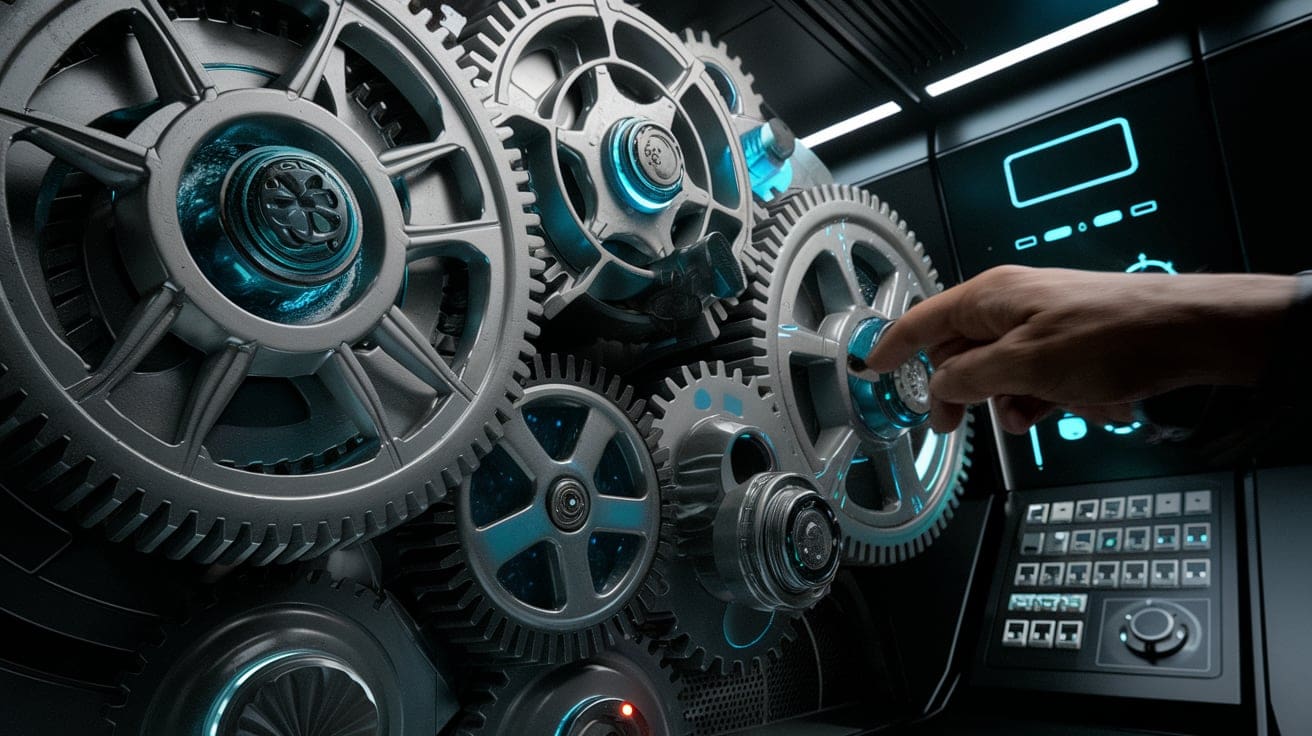
The best way to Carry out Scikit-learn Hyperparameter Optimization with Optuna
Picture by Creator | Ideogram
Introduction
Optuna is a machine studying framework particularly designed for automating hyperparameter optimization, that’s, discovering an externally fastened setting of machine studying mannequin hyperparameters that optimizes the mannequin’s efficiency. It may be seamlessly built-in with different machine studying modeling frameworks with Scikit-learn.
On this article, we present how one can mix each for a hyperparameter optimization job.
Performing Scikit-learn Hyperparameter Optimization with Optuna
If utilizing Optuna for the primary time in your Python growth atmosphere, you’ll have to set up it first.
On this instance, we are going to prepare a random forest classifier utilizing Scikit-learn’s digits
dataset, which is a “simplified model” of MNIST dataset for picture classification, containing 8×8 pixel photos of handwritten digits. Time to import the mandatory elements.
import optuna from sklearn.datasets import load_digits from sklearn.model_selection import train_test_split, cross_val_score from sklearn.ensemble import RandomForestClassifier |
Subsequent, we outline the primary operate to be referred to as later for conducting the hyperparameter optimization course of:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
def goal(trial): n_estimators = trial.suggest_int(“n_estimators”, 10, 200) max_depth = trial.suggest_int(“max_depth”, 2, 32, log=True) min_samples_split = trial.suggest_int(“min_samples_split”, 2, 10)
digits = load_digits() X_train, X_test, y_train, y_test = train_test_split(digits.knowledge, digits.goal, test_size=0.2, random_state=42)
clf = RandomForestClassifier( n_estimators=n_estimators, max_depth=max_depth, min_samples_split=min_samples_split, random_state=42 )
rating = cross_val_score(clf, X_train, y_train, cv=3, scoring=“accuracy”).imply() return rating |
As you possibly can observe, just about all the modeling course of is encapsulated on this goal(trial)
operate, which is a core operate managed by Optuna to automate the hyperparameter search, performing as many trials as specified within the operate’s argument. Let’s see the physique of the operate, i.e. the method outlined by us:
- Defining the hyperparameter search area or grid (see this article for a greater understanding of this idea). Hyperparameters within the search area are added to Optuna’s radar by way of the
suggest_in
operate. - Loading and splitting the dataset into coaching and take a look at subsets.
- Initializing the mannequin.
- Utilizing cross-validation to judge it.
Now we apply the two-step execution of the entire course of. First we outline the “research” or hyperparameter optimization experiment. Discover we set the path argument to “maximize” as a result of earlier we selected “accuracy” — the upper the higher — because the guiding metric for cross validation.
research = optuna.create_study(path=“maximize”) |
Second, the optimize methodology not directly invokes Optuna’s overridden goal operate, which is the primary operate we outlined earlier to encapsulate the method that should be trialled a number of occasions.
research.optimize(goal, n_trials=50) |
This can output a complete of fifty experiment studies, specifying the hyperparameter setting and ensuing mannequin’s accuracy for every trial. Since we’re excited by getting one of the best of all configurations tried, let’s simply get it:
print(“Greatest hyperparameters:”, research.best_params) print(“Greatest accuracy:”, research.best_value) |
And a pattern output:
Greatest hyperparameters: {‘n_estimators’: 188, ‘max_depth’: 17, ‘min_samples_split’: 4} Greatest accuracy: 0.9700765483646485 |
Implausible! Because of Optuna’s automated hyperparameter optimization capabilities, we discovered a random forest ensemble configuration able to classifying digit photos with over 97% prediction accuracy.
When you’ve got used Scikit-learn built-in courses for hyperparameter optimization like GridSearchCV
or RandomizedSearchCV
, you is perhaps questioning: why is Optuna higher? One motive is Optuna’s use of Bayesian optimization behind the scenes to make the hyperparameter tuning course of extra environment friendly. In addition to, Optuna applies inner methods like pruning or abruptly terminating unpromising trials, and helps search in additional advanced areas than standard strategies.
Wrapping Up
When you’ve got adopted together with the above, it’s best to now be capable of implement Scikit-learn hyperparameter optimization utilizing Optuna.
For extra info, try the next Machine Studying Mastery assets:
Source link