Language translation is likely one of the most necessary duties in pure language processing. On this tutorial, you’ll learn to implement a strong multilingual translation system utilizing the T5 (Textual content-to-Textual content Switch Transformer) mannequin and the Hugging Face Transformers library. By the tip of this tutorial, you’ll be capable of construct a production-ready translation system that may deal with a number of language pairs. Particularly, you’ll be taught:
- What’s the T5 mannequin and the way it works
- Find out how to generate a number of options for a translation
- Find out how to consider the standard of a translation
Let’s get began!
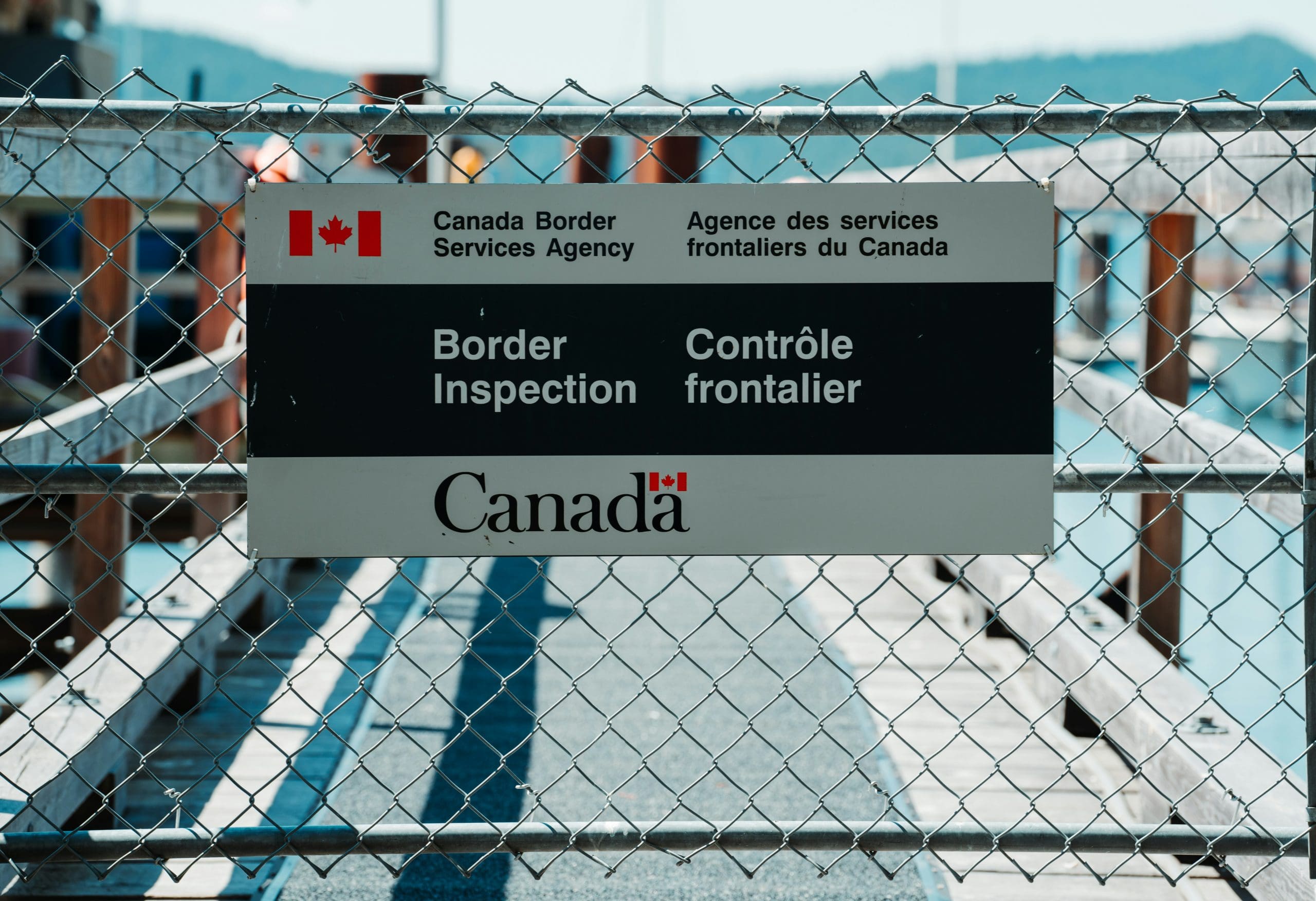
Implementing Multilingual Translation with T5 and Transformers
Hermes Rivera. Some rights reserved.
Overview
This publish is split into three components; they’re:
- Establishing the interpretation pipeline
- Translation with options
- High quality estimation
Setting Up the Translation Pipeline
Textual content translation is a basic process in pure language processing, and it impressed the invention of the unique transformer mannequin. T5, the Textual content-to-Textual content Switch Transformer, was launched by Google in 2020 and is a strong mannequin for translation duties as a consequence of its text-to-text method and pre-training on large multilingual datasets.
Textual content translation within the transformers
library is applied as “conditional technology”, which suggests the mannequin is producing textual content conditioned on the enter textual content, identical to a conditional likelihood distribution. Identical to all different fashions within the transformers
library, you’ll be able to instantiate a T5 mannequin in a number of traces of code. Earlier than you start, be sure to have the next dependencies put in:
pip set up torch transformers sentencepiece protobuf sacrebleu |
Let’s see easy methods to create a translation engine utilizing T5:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
import torch from transformers import T5ForConditionalGeneration, T5Tokenizer
class MultilingualTranslator: def __init__(self, model_name=“t5-base”): self.machine = torch.machine(“cuda” if torch.cuda.is_available() else “cpu”) print(f“Utilizing machine: {self.machine}”)
self.tokenizer = T5Tokenizer.from_pretrained(model_name, legacy=False) self.mannequin = T5ForConditionalGeneration.from_pretrained(model_name).to(self.machine)
def translate(self, textual content, source_lang, target_lang): “”“Translate textual content from supply language to focus on language”“” # Ensure the supply and goal languages are supported supported_lang = [“English”, “French”, “German”, “Spanish”] if source_lang not in supported_lang: increase ValueError(f“Unsupported supply language: {source_lang}”) if target_lang not in supported_lang: increase ValueError(f“Unsupported goal language: {target_lang}”) # Put together the enter textual content task_prefix = f“translate {source_lang} to {target_lang}” input_text = f“{task_prefix}: {textual content}” # Tokenize and generate translation inputs = self.tokenizer(input_text, return_tensors=“pt”, max_length=512, truncation=True) inputs = inputs.to(self.machine) outputs = self.mannequin.generate(**inputs, max_length=512, num_beams=4, length_penalty=0.6, early_stopping=True) # Decode and return translation translation = self.tokenizer.decode(outputs[0], skip_special_tokens=True) return translation
en_text = “Good day, how are you at the moment?” es_text = “¿Cómo estás hoy?” translator = MultilingualTranslator(“t5-base”)
translation = translator.translate(en_text, “English”, “French”) print(f“English: {en_text}”) print(f“French: {translation}”) print()
translation = translator.translate(en_text, “English”, “German”) print(f“English: {en_text}”) print(f“German: {translation}”) print()
translation = translator.translate(es_text, “Spanish”, “English”) print(f“Spanish: {es_text}”) print(f“English: {translation}”) |
The category MultilingualTranslator
instantiates a T5 mannequin and a tokenizer as common. The translate()
methodology is the place the precise translation magic occurs. You may see that it’s only a textual content technology with a immediate, and the immediate is solely saying, “translate X to Y”. As a result of it’s a textual content technology process, you’ll be able to see the parameters to manage the beam search, similar to num_beams
, length_penalty
, and early_stopping
.
The tokenizer units return_tensors="pt"
to return a PyTorch tensor, in any other case it would return a Python record of token IDs. You might want to try this as a result of the mannequin expects a PyTorch tensor. The default format of output will depend on the implementation of the tokenizer, therefore it’s good to seek the advice of the documentation to make use of it accurately.
The tokenizer is used once more after technology to decode the generated tokens again to textual content.
The output of the above code is:
Utilizing machine: cuda English: Good day, how are you at the moment? French: Bonjour, remark vous êtes-vous aujourd’hui?
English: Good day, how are you at the moment? German: Hallo, wie sind Sie heute?
Spanish: ¿Cómo estás hoy? English: Cómo estás hoy? |
You may see that the mannequin can translate from English to French or German, however didn’t translate from Spanish to English. It is a downside of the mannequin (in all probability associated to how the mannequin was skilled). You might have to strive one other mannequin to see if it really works higher.
Translation with Alternate options
Translating a sentence into a unique language is just not a one-to-one mapping. Due to the variation in grammar, phrase utilization, and sentence construction, there are a number of methods to translate a sentence.
Since textual content technology from the above mannequin makes use of beam search, you’ll be able to generate a number of options for a translation natively. You may modify the translate()
methodology to return a number of translations:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
def translate(self, textual content, source_lang, target_lang): “”“Translate textual content and report the beam search scores”“” supported_lang = [“English”, “French”, “German”, “Spanish”] if source_lang not in supported_lang: increase ValueError(f“Unsupported supply language: {source_lang}”) if target_lang not in supported_lang: increase ValueError(f“Unsupported goal language: {target_lang}”)
# Put together the enter textual content task_prefix = f“translate {source_lang} to {target_lang}” input_text = f“{task_prefix}: {textual content}” # Tokenize and generate translation inputs = self.tokenizer(input_text, return_tensors=“pt”, max_length=512, truncation=True) inputs = inputs.to(self.machine) with torch.no_grad(): outputs = self.mannequin.generate(**inputs, max_length=512, num_beams=4*4, num_beam_groups=4, num_return_sequences=4, diversity_penalty=0.8, length_penalty=0.6, early_stopping=True, output_scores=True, return_dict_in_generate=True) # Decode and return translation translation = [self.tokenizer.decode(output, skip_special_tokens=True) for output in outputs.sequences] return { “translation”: translation, “rating”: [float(score) for score in outputs.sequences_scores], } |
This modified methodology returns a dictionary with an inventory of translations and scores as an alternative of a single string of textual content. The mannequin’s output remains to be a tensor of logits, and it’s good to decode it again to textual content utilizing the tokenizer, one translation at a time.
The scores are used within the beam search. Therefore, they’re all the time in descending order, and the very best ones are cherry-picked for the output.
Let’s see how you should use it:
...
original_text = “This is a vital message that wants correct translation.” translator = MultilingualTranslator(“t5-base”) output = translator.translate(original_text, “English”, “French”) print(f“English: {original_text}”) print(“French:”) for textual content, rating in zip(output[“translation”], output[“score”]): print(f“- (rating: {rating:.2f}) {textual content}”) |
and the output is:
English: This is a vital message that wants correct translation. French: – (rating: -0.65) Il s’agit d’un message necessary qui a besoin d’une traduction précise. – (rating: -0.70) Il s’agit d’un message necessary qui doit être traduit avec précision. – (rating: -0.76) C’est un message necessary qui a besoin d’une traduction précise. – (rating: -0.81) Il s’agit là d’un message necessary qui doit être traduit avec précision. |
The scores are destructive as a result of they’re log chances. It’s best to use a extra advanced sentence to see the variations in translations.
High quality Estimation
The rating printed within the code above is the rating used within the beam search. It helps the auto-regressive technology full a sentence whereas sustaining variety. Think about that the mannequin is producing one token at a time, and every step emits a number of candidates. There are a number of paths to finish the sentence, and the variety of paths grows exponentially with the variety of auto-regressive steps explored. Beam search limits the variety of paths to trace by scoring every path and preserving the top-k paths solely.
Certainly you’ll be able to test the chances used within the technique of beam search. Within the mannequin, there’s a methodology compute_transition_scores()
that returns the transition scores of the generated tokens. You may strive it out as follows:
...
outputs = mannequin.generate(**inputs, max_length=512, num_beams=4*4, num_beam_groups=4, num_return_sequences=4, diversity_penalty=0.8, length_penalty=0.6, early_stopping=True, output_scores=True, return_dict_in_generate=True) transition_scores = mannequin.compute_transition_scores( outputs.sequences, outputs.scores, outputs.beam_indices, normalize_logits=True ) for idx, (out_tok, out_score) in enumerate(zip(outputs.sequences, transition_scores)): translation = tokenizer.decode(out_tok, skip_special_tokens=True) print(f“Translation: {translation}”) print(“token | token string | logits | likelihood”) for tok, rating in zip(out_tok[1:], out_score): print(f“| {tok:5d} | {tokenizer.decode(tok):14s} | {rating.numpy():.4f} | {np.exp(rating.numpy()):.2%}”) |
For a similar enter textual content because the earlier instance, the output of the above code snippet is:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
Translation: Il s’agit d’un message necessary qui a besoin d’une traduction précise. token | token string | logits | likelihood 802 | Il | -0.7576 | 46.88% 3 | | -0.0129 | 98.72% 7 | s | -0.0068 | 99.32% 31 | ‘ | -0.3295 | 71.93% 5356 | agit | -0.0033 | 99.67% 3 | | -0.3863 | 67.96% 26 | d | -0.0108 | 98.93% 31 | ‘ | -0.0005 | 99.95% 202 | un | -0.0152 | 98.49% 1569 | message | -0.0296 | 97.09% 359 | necessary | -0.0228 | 97.75% 285 | qui | -0.4194 | 65.74% 3 | | -0.9925 | 37.07% 9 | a | -0.1236 | 88.37% 6350 | besoin | -0.0114 | 98.87% 3 | | -0.1201 | 88.68% 26 | d | -0.0006 | 99.94% 31 | ‘ | -0.0007 | 99.93% 444 | une | -0.4557 | 63.40% 16486 | traduc | -0.0027 | 99.73% 1575 | tion | -0.0001 | 99.99% 17767 | précise | -0.6423 | 52.61% 5 | . | -0.0033 | 99.67% 1 | | -0.0006 | 99.94% Translation: Il s’agit d’un message necessary qui doit être traduit avec précision. token | token string | logits | likelihood 802 | Il | -0.7576 | 46.88% 3 | | -0.0129 | 98.72% … |
Within the for-loop, you print the token and the rating facet by facet. The primary token is all the time a padding token; therefore we match out_tok[1:]
with out_score
. The likelihood corresponds to the token at that step. It will depend on the earlier sequence of tokens, therefore the identical token could have completely different chances at completely different steps or completely different outputs sentences. A token with a excessive likelihood is probably going due to the grammar guidelines. A token with low likelihood means there are some doubtless options at that place. Notice that in beam search, the output is sampled from the probability-weighted distribution. Therefore the token you see from above is just not essentially the token with the very best likelihood generated.
There’s outputs.sequence_scores
, which is a normalized sum of the above chances within the outputs
object that accommodates the rating of every sequence. You should use it to estimate the standard of the interpretation.
Nevertheless, that is of little use to you since you aren’t implementing the beam search your self. The possibilities can’t inform you something concerning the high quality of the interpretation. You may’t examine them throughout completely different enter sentences, and you may’t examine them with completely different fashions.
One fashionable approach to estimate the standard of a translation is to make use of the BLEU (Bilingual Evaluation Understudy) score. You should use the sacrebleu
library to compute the BLEU rating of a translation, however you will want a reference translation for the rating. Beneath is an instance:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
... import sacrebleu
sample_document = “”“ Machine translation has developed considerably through the years. Early methods used rule-based approaches that outlined grammatical guidelines for languages. Statistical machine translation later emerged, utilizing giant corpora of translated texts to be taught translation patterns robotically. ““” reference_translation = “”“ La traduction automatique a considérablement évolué au fil des ans. Les premiers systèmes utilisaient des approches basées sur des règles définissant les règles grammaticales des langues. La traduction automatique statistique est apparue plus tard, utilisant de vastes corpus de textes traduits pour apprendre automatiquement des modèles de traduction. ““”
translator = MultilingualTranslator(“t5-base”) output = translator.translate(sample_document, “English”, “French”) print(f“English: {sample_document}”) print(“French:”) for textual content, rating in zip(output[“translation”], output[“score”]): bleu = sacrebleu.corpus_bleu([text], [[reference_translation]]) print(f“- (rating: {rating:.2f}, bleu: {bleu.rating:.2f}) {textual content}”) |
The output could also be:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
English: Machine translation has developed considerably through the years. Early methods used rule-based approaches that outlined grammatical guidelines for languages. Statistical machine translation later emerged, utilizing giant corpora of translated texts to be taught translation patterns robotically.
French: – (rating: -0.94, bleu: 26.49) La traduction automatique a beaucoup évolué au fil des ans. Les premiers systèmes utilisaient des approches fondées sur des règles qui définissaient des règles grammaticales pour les langues. – (rating: -1.26, bleu: 56.78) La traduction automatique a beaucoup évolué au fil des ans. Les premiers systèmes utilisaient des approches fondées sur des règles qui définissaient des règles grammaticales pour les langues. La traduction automatique statistique s’est développée plus tard, en utilisant de vastes corpus de textes traduits pour apprendre automatiquement les schémas de traduction. – (rating: -1.26, bleu: 56.41) La traduction automatique a beaucoup évolué au fil des ans. Les premiers systèmes utilisaient des approches fondées sur des règles qui définissaient des règles grammaticales pour les langues. La traduction automatique statistique a ultérieurement vu le jour, utilisant de vastes corpus de textes traduits pour apprendre automatiquement les schémas de traduction. – (rating: -1.32, bleu: 53.79) La traduction automatique a beaucoup évolué au fil des ans. Les premiers systèmes utilisaient des approches fondées sur des règles qui définissaient des règles grammaticales pour les langues. La traduction automatique statistique a ultérieurement vu le jour, en utilisant de vastes corpus de textes traduits pour apprendre automatiquement les modes de traduction. |
The BLEU rating exhibits how intently the interpretation matches the reference. It ranges from 0 to 100; the upper the rating, the higher. You may see that the mannequin’s scoring of the translations doesn’t match the BLEU rating. On one hand, this highlights that the rating is to not consider the standard of the interpretation. Alternatively, this will depend on the reference translation you present.
Additional Readings
Beneath are some sources that you could be discover helpful:
Abstract
On this tutorial, you’ve constructed a complete multilingual translation system utilizing T5 and the Transformers library. Particularly, you’ve discovered:
- Find out how to implement a fundamental translation system utilizing T5 mannequin and a immediate
- Find out how to modify the beam search to generate a number of options for a translation
- Find out how to estimate the standard of a translation utilizing BLEU rating
Source link