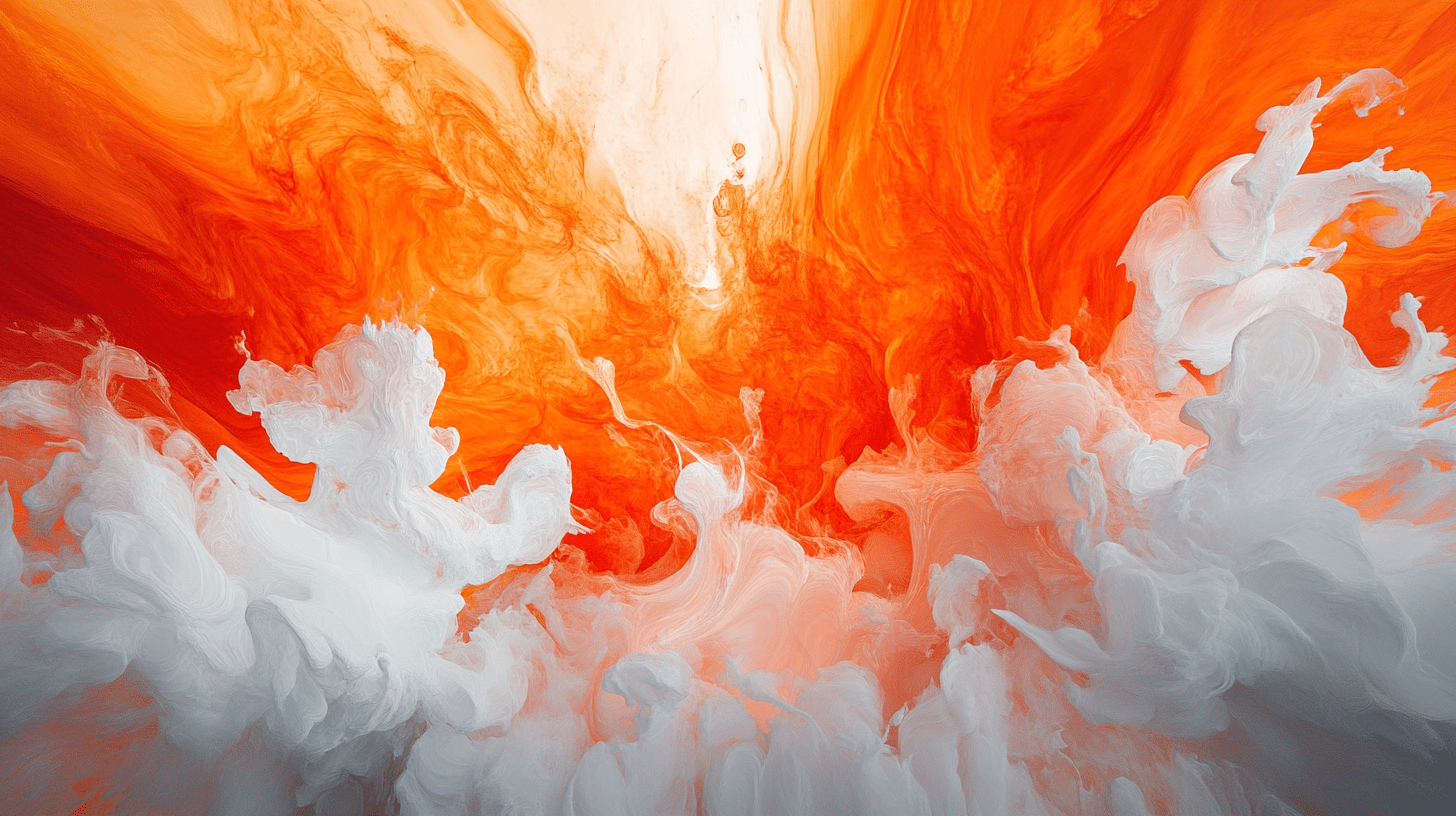
Integrating TensorFlow and NumPy for Customized Operations
Picture by Editor | Midjourney
Combining the facility of TensorFlow and NumPy creates a bridge between high-performance machine studying and the precision of numerical computing. This integration is a game-changer for machine studying builders in search of to push boundaries, providing unparalleled flexibility for creating customized operations, optimizing workflows, and remodeling how numerical information is processed and modeled. By mixing TensorFlow’s {hardware} acceleration with NumPy’s wealthy mathematical toolkit, a world of modern potentialities unfolds for tackling advanced computational challenges.
Whereas TensorFlow offers its personal tensor operations much like NumPy’s, there are a number of eventualities where combining the two libraries proves advantageous:
- Leverage present NumPy code: Many scientific computing workflows and legacy codebases depend on NumPy. TensorFlow’s integration permits for seamless incorporation of such workflows into machine studying pipelines.
- Customized operations: NumPy’s huge array of mathematical features can increase TensorFlow’s capabilities, enabling the creation of customized operations without having to implement them from scratch.
- Effectivity: TensorFlow optimizes computations on GPUs and TPUs, providing a significant speed-up for NumPy-based operations when transitioned to TensorFlow tensors.
- Interoperability: TensorFlow natively helps interoperability with NumPy, permitting tensors and arrays to be interchanged with minimal effort.
Key Options of TensorFlow-NumPy Interoperability
TensorFlow’s NumPy API (tf.experimental.numpy
) provides a near-identical expertise to plain NumPy, making it simpler to carry out operations on TensorFlow tensors as if they have been NumPy arrays. Key highlights embrace:
- TensorFlow tensors as drop-in replacements: TensorFlow tensors can be utilized instead of NumPy arrays in most mathematical operations.
- Computerized differentiation: Operations carried out utilizing
tf.experimental.numpy
are differentiable, enabling gradient-based optimization workflows. - Keen execution compatibility: NumPy features in TensorFlow assist keen execution, offering speedy suggestions throughout code growth and debugging.
Setting Up Your Surroundings
Be certain that each TensorFlow and NumPy are put in in your atmosphere. Use the next instructions to put in or improve the libraries:
pip set up tensorflow numpy —improve |
Confirm the installations by importing the libraries in Python:
import tensorflow as tf import numpy as np print(tf.__version__) print(np.__version__) |
Having the newest variations ensures compatibility and access to the newest features in each libraries.
Utilizing NumPy Arrays in TensorFlow
NumPy arrays will be straight transformed to TensorFlow tensors utilizing the tf.convert_to_tensor
operate. Conversely, TensorFlow tensors will be transformed again to NumPy arrays utilizing the .numpy()
methodology. This two-way conversion types the spine of seamless interoperability.
Instance: Conversion Between NumPy and TensorFlow
# Create a NumPy array np_array = np.array([1.0, 2.0, 3.0])
# Convert to TensorFlow tensor tf_tensor = tf.convert_to_tensor(np_array)
# Carry out a TensorFlow operation result_tensor = tf_tensor * 2
# Convert again to NumPy result_array = result_tensor.numpy() print(“Unique NumPy array:”, np_array) print(“TensorFlow tensor:”, tf_tensor) print(“Outcome as NumPy array:”, result_array) |
Output:
Unique NumPy array: [1. 2. 3.] TensorFlow tensor: tf.Tensor([1. 2. 3.], form=(3,), dtype=float32) Outcome as NumPy array: [2. 4. 6.] |
Customized Operations with TensorFlow and NumPy
Customized operations typically require mathematical computations not natively out there in TensorFlow. In such circumstances, NumPy offers a wealthy set of instruments for implementing the specified performance.
Let’s check out a number of examples illustrating find out how to mix the strengths of each libraries.
Instance 1: Implementing a Customized Activation Operate
Suppose you need to implement a customized activation operate utilizing NumPy operations:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
def custom_activation(x): # Use NumPy for mathematical operations return np.log1p(np.exp(x)) # Easy approximation of ReLU
# Enter TensorFlow tensor input_tensor = tf.fixed([–1.0, 0.0, 1.0, 2.0], dtype=tf.float32)
# Convert TensorFlow tensor to NumPy array input_array = input_tensor.numpy()
# Apply customized activation output_array = custom_activation(input_array)
# Convert again to TensorFlow tensor output_tensor = tf.convert_to_tensor(output_array) print(“Enter tensor:”, input_tensor) print(“Output tensor:”, output_tensor) |
Output:
Enter tensor: tf.Tensor([–1. 0. 1. 2.], form=(4,), dtype=float32) Output tensor: tf.Tensor([0.3133 0.6931 1.3133 2.1269], form=(4,), dtype=float32) |
This instance demonstrates the benefit with which customized mathematical features will be built-in into TensorFlow workflows, leveraging NumPy’s intensive capabilities.
Instance 2: Customized Loss Operate for Optimization
Customized loss features are important in machine studying workflows. Right here’s an instance combining TensorFlow and NumPy for a customized loss:
def custom_loss(y_true, y_pred): # Calculate squared error utilizing NumPy return np.sum(np.sq.(y_true – y_pred))
# True and predicted values y_true = tf.fixed([1.0, 2.0, 3.0], dtype=tf.float32) y_pred = tf.fixed([1.1, 1.9, 3.2], dtype=tf.float32)
# Convert to NumPy arrays true_array = y_true.numpy() pred_array = y_pred.numpy()
# Compute loss loss_value = custom_loss(true_array, pred_array) print(“Customized loss worth:”, loss_value) |
Output:
By integrating NumPy into TensorFlow, builders achieve entry to a well-known and intensive toolkit for implementing advanced loss features.
Optimizing NumPy-Based mostly Operations in TensorFlow
For prime-performance computing, it’s essential to leverage TensorFlow’s hardware acceleration whereas retaining NumPy’s flexibility:
Instance: Wrapping NumPy Code in tf.operate
def compute_with_numpy(x): # Convert tensor to NumPy array x_np = x.numpy()
# Carry out NumPy operations result_np = np.exp(x_np) + np.log1p(x_np)
# Convert again to TensorFlow tensor return tf.convert_to_tensor(result_np)
# Enter tensor input_tensor = tf.fixed([0.1, 0.2, 0.3], dtype=tf.float32)
# Compute end result end result = compute_with_numpy(input_tensor) print(“Outcome tensor:”, end result) |
Output:
Outcome tensor: tf.Tensor([1.1051709 1.2214028 1.3498588], form=(3,), dtype=float32) |
Superior Use Circumstances
The seamless integration of TensorFlow and NumPy additionally permits extra superior use circumstances, together with:
- Hybrid Modeling: Develop workflows the place preprocessing is done in NumPy whereas the mannequin coaching leverages TensorFlow. For example, reworking datasets utilizing NumPy’s matrix operations earlier than feeding them to a TensorFlow mannequin.
- Scientific Computing: Conduct scientific simulations in NumPy, utilizing TensorFlow to optimize parameters or run simulations on GPUs. This mixture bridges scientific computing and machine studying.
- Automated Differentiation: Utilizing
tf.experimental.numpy
, operations carried out on tensors routinely achieve gradient assist, enabling machine studying duties with NumPy-like syntax whereas using TensorFlow’s optimization capabilities.
Conclusion
With the mixture of TensorFlow’s {hardware} acceleration and machine studying capabilities with NumPy’s sturdy mathematical toolkit, builders can construct refined workflows tailor-made to their particular wants. Understanding and leveraging the interaction between these libraries will open the door to extra modern options in computational science and synthetic intelligence.
Whereas TensorFlow and NumPy integration is highly effective, you’ll be able to additional improve efficiency when you hold the next efficiency concerns in thoughts:
- Keep away from frequent conversions: Decrease switching between TensorFlow tensors and NumPy arrays to forestall pointless overhead.
- Leverage TensorFlow operations: Use TensorFlow’s native operations each time doable for GPU/TPU acceleration.
- Batch operations: Course of information in batches to completely make the most of {hardware} sources.
Whether or not you’re creating machine studying fashions, conducting simulations, or crafting customized operations, the TensorFlow-NumPy synergy offers a unified and highly effective framework for tackling advanced computational challenges.
Source link