Transformer fashions are the usual fashions to make use of for NLP duties at present. Virtually all the NLP duties contain producing textual content however it isn’t the direct output of the mannequin. You could count on the mannequin that can assist you generate textual content that’s coherent and contextually related. Whereas partially that is associated to the standard of the mannequin, the era parameters additionally play a vital position within the high quality of the generated textual content.
On this put up, you’ll discover the important thing parameters that management textual content era in transformer fashions. You will note how these parameters have an effect on the standard of the generated textual content and how you can tune them for various purposes. Specifically, you’ll study:
- The core parameters that management textual content era in transformer fashions
- The totally different decoding methods
- Learn how to management the creativity and coherence of generated textual content
- Learn how to fine-tune era parameters for particular purposes
Let’s get began!
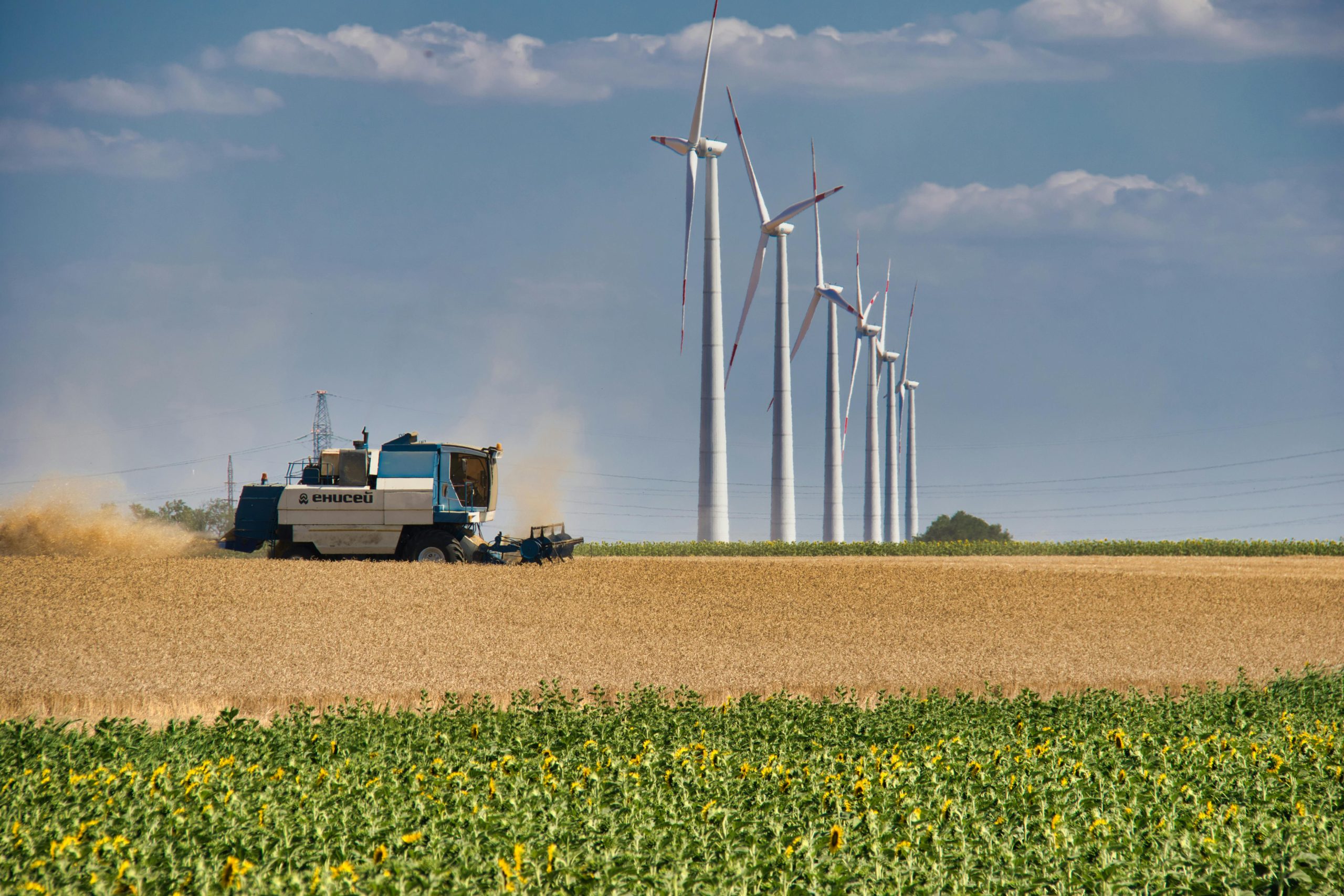
Understanding Textual content Era Parameters in Transformers
Picture by Anton Klyuchnikov. Some rights reserved.
Overview
This put up is split into seven components; they’re:
- Core Textual content Era Parameters
- Experimenting with Temperature
- High-Ok and High-P Sampling
- Controlling Repetition
- Grasping Decoding and Sampling
- Parameters for Particular Purposes
- Beam Search and A number of Sequences Era
Core Textual content Era Parameters
Let’s choose the GPT-2 mannequin for example. It’s a small transformer mannequin that doesn’t require loads of computational assets however continues to be able to producing high-quality textual content. A easy instance to generate textual content utilizing the GPT-2 mannequin is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
# create mannequin and tokenizer tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
# tokenize enter immediate to sequence of ids immediate = “Synthetic intelligence is” inputs = tokenizer(immediate, return_tensors=“pt”) # generate output as a sequence of token ids output = mannequin.generate( **inputs, max_length=50, num_return_sequences=1, temperature=1.0, top_k=50, top_p=1.0, repetition_penalty=1.0, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) # convert token ids into textual content strings generated_text = tokenizer.decode(output[0], skip_special_tokens=True)
print(f“Immediate: {immediate}”) print(“Generated Textual content:”) print(generated_text) |
When you run this code, you might even see:
Immediate: Synthetic intelligence is Generated Textual content: Synthetic intelligence is used within the manufacturing of know-how, the supply of which is set by technological change. For instance, an autonomous automobile can change its steering wheel to assist keep away from driving visitors. Within the case of synthetic intelligence, this could change what customers |
You offered a immediate of solely three phrases, and the mannequin generated a protracted piece of textual content. This isn’t generated in a single shot, however the mannequin is invoked a number of occasions in an iterative course of.
You’ll be able to see the quite a few parameters used within the generate()
perform. The primary one you used is max_length
. Trivially, this controls how lengthy the generated textual content needs to be, in variety of tokens. Often, the mannequin is producing one token at a time utilizing the immediate as context. Then, append the newly generated token to the immediate and generate the following token. Subsequently, the longer you need the generated textual content to be, the extra time it takes to generate it. Be aware that it’s tokens in concern, not phrases, since you used a subword tokenizer with the GPT-2 mannequin. One token could also be only a subword unit, not a full phrase.
Nonetheless, the mannequin shouldn’t be producing any single token particularly. As a substitute, it’s producing a “logit”, which is a vector of possibilities of the following token. The logit is a protracted vector, precisely so long as the scale of the vocabulary. Given it’s a chance distribution over all of the doable “subsequent tokens”, you possibly can choose the token with the best chance (while you set do_sample=False
), or another token with non-zero chance (while you set do_sample=True
). That is what all different parameters are for.
The temperature
parameter skews the chance distribution. A decrease temperature emphasizes the most probably token, whereas a better temperature diminishes the distinction between a possible and unlikely token. The default temperature is 1.0, and it needs to be a optimistic worth. The top_k
parameter then selects solely the highest $ok$ tokens relatively than the whole vocabulary of tokens. Then the chance is recalculated to sum to 1. Subsequent, if top_p
is about, this set of $ok$ tokens is additional filtered to maintain the highest ones that make up the overall chance of $p$. This remaining set of tokens is then used to pattern the following token, and this course of is known as the nucleus sampling.
Keep in mind that you’re producing a sequence of tokens, one by one. Chances are high that you will note the identical token repeatedly in each step, and you might even see the identical token produced within the sequence. It’s often not what you need, so it’s possible you’ll need to lower the chance of these tokens while you see them once more. That’s what the repetition_penalty
parameter is for.
Experimenting with Temperature
Given what the assorted parameters do, let’s see how the output modifications while you modify a few of them.
The temperature parameter has a big affect on the creativity and randomness of the generated textual content. You’ll be able to see its impact with the next instance:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
immediate = “The way forward for synthetic intelligence is” inputs = tokenizer(immediate, return_tensors=“pt”)
# Generate textual content with totally different temperature values temperatures = [0.2, 0.5, 1.0, 1.5] print(f“Immediate: {immediate}”) for temp in temperatures: print() print(f“Temperature: {temp}”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=temp, top_k=50, top_p=1.0, repetition_penalty=1.0, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text) |
Once you run this code, you might even see:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
Immediate: The way forward for synthetic intelligence is
Temperature: 0.2 Generated Textual content: The way forward for synthetic intelligence is unsure. The way forward for synthetic intelligence is unsure.
The way forward for synthetic intelligence is unsure. The way forward for synthetic intelligence is unsure.
The way forward for synthetic intelligence is unsure. The way forward for synthetic intelligence is unsure.
The way forward for synthetic intelligence is unsure. The way forward for synthetic intelligence is unsure.
The way forward for synthetic intelligence is unsure. The way forward for synthetic intelligence is unsure.
The way forward for synthetic intelligence is unsure. The long run
Temperature: 0.5 Generated Textual content: The way forward for synthetic intelligence is unsure.
“There may be loads of work to be finished on this,” stated Eric Schmitt, a professor of pc science and engineering on the College of California, Berkeley.
“We’re on the lookout for a method to make AI extra like computer systems. We have to take a step again and take a look at how we give it some thought and the way we work together with it.”
Schmitt stated he is assured that synthetic intelligence will ultimately be capable of do greater than
Temperature: 1.0 Generated Textual content: The way forward for synthetic intelligence shouldn’t be but clear, nevertheless.”
“Is the method that we try to do via pc imaginative and prescient and the power to take a look at an individual at a number of factors with none lack of intelligence attributable to not seeing an individual at a number of factors?” requested Richard. “I additionally assume the individuals who are doing this analysis are extraordinarily attention-grabbing to me attributable to with the ability to see people at a spread of various cut-off dates. Specifically, they’ve proven how to do a reasonably complicated
Temperature: 1.5 Generated Textual content: The way forward for synthetic intelligence is an period to recollect as a lot as Google in search outcomes, notably ones not supported by a lot else for some years — and it would appear like the search large is now simply nearly as good with out synthetic intelligence. [Graphic image from Shutterstock] |
With a low temperature (e.g., 0.2), the textual content turns into extra targeted and deterministic, usually sticking to frequent phrases and traditional concepts. You additionally see that it retains repeating the identical sentence as a result of the chance is focused on a couple of tokens, limiting range. This may be resolved by utilizing the repetition penalty parameter that’s lined in a piece beneath.
With a medium temperature (e.g., 0.5 to 1.0), the textual content has a very good stability of coherence and creativity. The generated textual content might not be factual, however the language is pure.
With a excessive temperature (e.g., 1.5), the textual content turns into extra random and inventive, however can also be much less coherent and typically illogical. The language could also be obscure, similar to the instance above.
Selecting the best temperature relies on your software. In case you are making a helper for code completion or writing, a decrease temperature is usually higher. For inventive writing or brainstorming, a better temperature can produce extra various and attention-grabbing outcomes.
High-Ok and High-P Sampling
The nucleus sampling parameters management how versatile you enable the mannequin to select the following token. Do you have to modify the top_k
parameter or the top_p
parameter? Let’s see their impact in an instance:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
immediate = “The easiest way to study programming is” inputs = tokenizer(immediate, return_tensors=“pt”)
# Generate textual content with totally different top_k values top_k_values = [5, 20, 50] print(f“Immediate: {immediate}”)
for top_k in top_k_values: print() print(f“High-Ok = {top_k}”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=1.0, top_k=top_k, top_p=1.0, repetition_penalty=1.0, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text)
# Generate textual content with totally different top_p values top_p_values = [0.5, 0.7, 0.9] for top_p in top_p_values: print() print(f“High-P = {top_p}”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=1.0, top_k=0, top_p=top_p, repetition_penalty=1.0, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text) |
Once you run this code, you might even see:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
Immediate: The easiest way to study programming is
High-Ok = 5 Generated Textual content: The easiest way to study programming is to have the ability to study the fundamentals in a really quick period of time, after which study to make use of them successfully and rapidly.
If you wish to be a profitable programmer on this approach, you must study to make use of the strategies within the above video to study the fundamentals of programming.
If you wish to study to code extra successfully, you too can get extra skilled programmers by doing the next:
Studying to Code
Studying to code may be very
High-Ok = 20 Generated Textual content: The easiest way to study programming is to study it.
As a way to get began with Ruby you are going to need to make a couple of errors, some of them will be pretty apparent.
To begin with, you are going to have to put in writing a perform that takes in a worth. What this implies is that you will make a brand new occasion of the Ruby perform. You’ll be able to learn extra about this in Half 1 of this course, or simply attempt it out from the REPL.
High-Ok = 50 Generated Textual content: The easiest way to study programming is to change into acquainted with the language and the software program. One of many first and commonest types of programming is to create, modify, and distribute code.
Nonetheless, there are only a few programming libraries that may present us with all that we want.
The next pattern programming program makes use of a number of the above, however doesn’t present one of the best ways to study programming. It was written in Java and in C or C++.
The unique supply code is
High-P = 0.5 Generated Textual content: The easiest way to study programming is to have the ability to create a device for you. That is what I do.
That is why I am right here at present.
I am right here to speak in regards to the fundamentals of programming, and I will inform you how you can study programming.
I am right here to speak about studying programming.
It is easy to overlook that you do not have to know how you can program. It is easy to overlook that you do not have to know the way
High-P = 0.7 Generated Textual content: The easiest way to study programming is to follow programming. Study the ideas of programming by observing and performing workouts.
I used to work in a world of data which included all kinds of issues, and was capable of make amends for them and perceive them from their perspective. For example, I discovered to perk up and do 5 squats. Then, I must follow some kind of overhead coaching. I might attempt to study the most effective approach and add that to my repertoire.
What
High-P = 0.9 Generated Textual content: The easiest way to study programming is to change into a very good hacker. Do not use any programming instruments. Only a common dot-com consumer, an occasional coding learner, and keep it up.
— Victoria E. Nichols |
You’ll be able to see that with a small $ok$ worth, akin to 5, the mannequin has fewer choices to select from, leading to extra predictable textual content. On the excessive, when $ok=1$, the mannequin all the time picks the one token with the best chance, which is grasping decoding, and sometimes produces poor output. With a bigger $ok$, akin to 50, the mannequin has extra choices to select from, leading to extra various textual content.
Equally, for the top_p
parameter, a smaller $p$ means the mannequin selects from a smaller set of high-probability tokens, leading to extra targeted textual content. With a bigger $p$, akin to 0.9, the mannequin has a wider choice, doubtlessly resulting in extra assorted textual content. Nonetheless, what number of choices it’s possible you’ll choose for a given $p$ shouldn’t be fastened. It relies on the chance distribution because the mannequin predicted. When the mannequin may be very assured in regards to the subsequent token (akin to restricted by some grammar guidelines), solely a really small set of tokens is allowed. This adaptive nature can also be why top-p sampling is usually most well-liked over top-k sampling.
Controlling Repetition
Repetition is a typical concern in textual content era. The repetition_penalty
parameter helps handle this by penalizing tokens which have already appeared within the generated textual content. Let’s see the way it works:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
immediate = “As soon as upon a time, there was a” inputs = tokenizer(immediate, return_tensors=“pt”)
# Generate textual content with totally different repetition penalties penalties = [1.0, 1.2, 1.5, 2.0] print(f“Immediate: {immediate}”) for penalty in penalties: print() print(f“Repetition penalty: {penalty}”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=0.3, top_k=50, top_p=1.0, repetition_penalty=penalty, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text) |
Once you run this code, you might even see:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
Immediate: As soon as upon a time, there was a
Repetition penalty: 1.0 Generated Textual content: As soon as upon a time, there was a substantial amount of confusion about what was happening. The very first thing that got here to thoughts was the truth that the federal government had already been in place for a very long time, and that the federal government had been in place for a very long time. And it was clear that the federal government had been in place for a very long time. And it was clear that the federal government had been in place for a very long time. And it was clear that the federal government had been in place for a protracted
Repetition penalty: 1.2 Generated Textual content: As soon as upon a time, there was a substantial amount of speak in regards to the chance that this can be a chance for us to see extra and higher issues in our lives. We had been speaking on Fb all day lengthy with individuals who have been taken with what we might do subsequent or how they could assist others discover their very own approach out.” “We have all the time needed to ensure everybody has entry,” he continued; “nevertheless it’s not like you possibly can simply go into your room at night time trying round with out seeing
Repetition penalty: 1.5 Generated Textual content: As soon as upon a time, there was a person who had been known as to the service of God. He got here and stated: “I’m an apostle from Jerusalem.” And he answered him with nice pleasure, saying that it isn’t doable for me now on this life with out having obtained Jesus Christ as our Lord; however I might be saved via Him alone as a result of my Father has despatched Me into all issues by His Holy Spirit (John 1). The Christian Church teaches us how way more than another faith can
Repetition penalty: 2.0 Generated Textual content: As soon as upon a time, there was a person who had been despatched to town of Nausicaa by his father. The king’s son and brother have been killed in battle at that place; however when he returned with them they discovered him lifeless on their approach again from war-time.[1] The King gave orders for an expedition in opposition to this unusual creature known as “the Gorgon,” which got here out into area throughout one night time after it attacked Earth[2]. It is alleged that these creatures |
Within the code above, temperature is about to 0.3 to emphasise the impact of the repetition penalty. With a low penalty of 1.0, you possibly can see that the mannequin repeats the identical phrase again and again. The mannequin would possibly simply get caught in loops when the opposite settings restrict the candidate tokens to a small subset. However at a excessive penalty, akin to 2.0 or above, the mannequin strongly avoids repetition, which may typically result in much less pure textual content. A average penalty (e.g., 1.2 to 1.5) is usually a very good compromise to keep up coherence.
In spite of everything, the parameters to set within the generate()
perform is to maintain the textual content stream naturally. You could need to modify these parameters by experimentation to see which appears greatest on your specific software. Be aware that these parameters could depend upon the mannequin you might be utilizing, since every mannequin could generate tokens with a distinct distribution.
Grasping Decoding and Sampling
The do_sample parameter controls whether or not the mannequin makes use of sampling (probabilistic collection of tokens) or grasping decoding (all the time deciding on probably the most possible token). Let’s evaluate these approaches:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
immediate = “The key to happiness is” inputs = tokenizer(immediate, return_tensors=“pt”)
# Generate textual content with grasping decoding vs. sampling print(f“Immediate: {immediate}n”) print(“Grasping Decoding (do_sample=False):”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=1.0, top_k=50, top_p=1.0, repetition_penalty=1.0, do_sample=False, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text) print() print(“Sampling (do_sample=True):”) output = mannequin.generate( **inputs, max_length=100, num_return_sequences=1, temperature=1.0, top_k=50, top_p=1.0, repetition_penalty=1.0, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) generated_text = tokenizer.decode(output[0], skip_special_tokens=True) print(“Generated Textual content:”) print(generated_text) |
Strive working this code a number of occasions and observing the output. You’ll discover that the output of grasping decoding is all the time the identical, whereas the output of sampling is totally different every time. Grasping decoding is deterministic for a hard and fast immediate. The mannequin generates a chance distribution, and probably the most possible token is chosen. No randomness is concerned. The output is extra prone to be repetitive and never helpful.
The sampling output is stochastic as a result of the output tokens are chosen primarily based on the mannequin’s predicted chance distribution. The randomness permits the mannequin to generate extra various and inventive textual content whereas the output continues to be coherent so long as the opposite era parameters are set correctly. Within the case of sampling output, you possibly can set num_return_sequences
to a quantity higher than 1 to generate a number of sequences in parallel for a similar immediate. This parameter is meaningless for grasping decoding.
Parameters for Particular Purposes
For a specific software, what parameter values do you have to set? There isn’t any concrete reply. You absolutely must run some experiments to seek out the most effective mixtures. However it’s possible you’ll use the next as a place to begin:
- Factual Era:
- Decrease
temperature
(0.2 to 0.4) for extra deterministic output - Average
top_p
(0.8 to 0.9) to filter out unlikely tokens - Increased
repetition_penalty
(1.2 to 1.5) to keep away from repetitive statements
- Decrease
- Inventive Writing:
- Increased
temperature
(1.0 to 1.3) for extra inventive and various output - Increased
top_p
(0.9 to 0.95) to permit for extra potentialities - Decrease
repetition_penalty
(1.0 to 1.1) to permit some stylistic repetition
- Increased
- Code Era:
- Decrease
temperature
(0.1 to 0.3) for extra exact and proper code - Decrease
top_p
(0.7 to 0.8) to give attention to the most probably tokens - Increased
repetition_penalty
(1.3 to 1.5) to keep away from redundant code
- Decrease
- Dialogue Era:
- Average
temperature
(0.6 to 0.8) for pure however targeted responses - Average
top_p
(0.9) for a very good stability of creativity and coherence - Average
repetition_penalty
(1.2) to keep away from repetitive phrases
- Average
Keep in mind that the language mannequin shouldn’t be an ideal oracle. It could make errors. The above parameters are that can assist you match the era course of to the anticipated model of the output, however to not assure the correctness. The output you get could comprise errors.
Beam Search and A number of Sequences Era
Within the above examples, the era course of is autoregressive. It’s an iterative course of that generates one token at a time.
Since every step generates one token via sampling, nothing prevents you from producing a number of tokens directly. When you try this, you’ll generate a number of output sequences for one enter immediate. Theoretically, when you generate $ok$ tokens at every step and also you set the size to return as $n$, you’ll generate $ok^n$ sequences. This is usually a large quantity, and it’s possible you’ll need to restrict this to just a few.
The primary method to generate a number of sequences is to set num_return_sequences
to a quantity $ok$. You generate $ok$ tokens in step one. Then full the sequence for every of them. This basically duplicated the immediate $ok$ occasions within the era.
The second approach is to make use of beam search. It’s a extra refined method to generate a number of sequences. It retains observe of probably the most promising sequences and explores them in parallel. As a substitute of producing $ok^n$ sequences to overwhelm the reminiscence, it retains solely $ok$ greatest sequences at every step. Every token era step will develop this set quickly and prune it again to $ok$ greatest sequences.
To make use of beam search, it’s good to set num_beams
to a quantity $ok$. Every step will develop every of the $ok$ sequences for yet another token, ensuing $ok^2$ sequences, after which choose the most effective $ok$ sequences to proceed to the following step. You may additionally set early_stopping=True
to cease the era when the top of the sequence is reached. You must also set num_return_sequences
to restrict the ultimate choice on the output.
The collection of a sequence is often primarily based on the cumulative chance of the tokens within the sequence. However you may additionally skew the choice by different standards, akin to including a size penalty or avoiding repeating n-grams. Under is an instance of utilizing beam search:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
import torch from transformers import GPT2LMHeadModel, GPT2Tokenizer
tokenizer = GPT2Tokenizer.from_pretrained(“gpt2”) mannequin = GPT2LMHeadModel.from_pretrained(“gpt2”)
immediate = “The important thing to profitable machine studying is” inputs = tokenizer(immediate, return_tensors=“pt”)
# Generate textual content with grasping decoding vs. sampling print(f“Immediate: {immediate}n”) outputs = mannequin.generate( **inputs, num_beams=5, # Variety of beams to make use of early_stopping=True, # Cease when all beams have completed no_repeat_ngram_size=2, # Keep away from repeating n-grams num_return_sequences=3, # Return a number of sequences max_length=100, temperature=1.5, do_sample=True, pad_token_id=tokenizer.eos_token_id, ) for idx, output in enumerate(outputs): generated_text = tokenizer.decode(output, skip_special_tokens=True) print(f“Generated Textual content ({idx+1}):”) print(generated_text) |
You could add extra era parameters (akin to length_penalty
) to manage the era course of. The instance above set a better temperature to spotlight the output of beam search. When you run this code, you might even see:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
Immediate: The important thing to profitable machine studying is
Generated Textual content (1): The important thing to profitable machine studying is to have the ability to study from the world round you. It’s our job to ensure that we’re studying from individuals, relatively than simply from machines.
So, let’s take a step again and take a look at how we are able to study. Here is an inventory of the instruments we use to assist us try this. We will go over a couple of of them right here and provide you with a basic concept of what they’re and the way you should utilize them to create
Generated Textual content (2): The important thing to profitable machine studying is to have the ability to study from the world round you. It’s our job to ensure that we’re studying from individuals, relatively than simply from machines.
So, let’s take a step again and take a look at how we are able to study. Here is an inventory of the instruments we use to assist us try this. We will go over a couple of of them right here and provide you with a basic concept of what they’re and the way you should utilize them and what
Generated Textual content (3): The important thing to profitable machine studying is to have the ability to study from the world round you. It’s our job to ensure that we’re studying from individuals, relatively than simply from machines.
So, let’s take a step again and take a look at how we are able to study. Here is an inventory of the instruments we use to assist us try this. We will go over a couple of of them right here and provide you with a basic concept of what they’re and the way they work. You need to use |
The variety of output sequences continues to be managed by num_return_sequences,
however the course of to generate them makes use of the beam search algorithm. It’s not straightforward to establish whether or not beam search is used from the output. One signal is that the output of beam search shouldn’t be as various as simply setting num_return_sequences
since many extra sequences are generated, and people with larger cumulative possibilities are chosen. This filtering certainly lowered the variety of the output.
Additional Readings
Under are some additional readings that you could be discover helpful:
Abstract
On this put up, you see how the various parameters within the generate()
perform can be utilized to manage the era course of. You’ll be able to modify these parameters to make the output match the model you’ll count on on your software. Particularly, you discovered:
- Learn how to use temperature to manage the chance distribution of the output
- Learn how to use top-k and top-p to manage the variety of the output
- Learn how to management output utilizing repetition penalty, beam search, and grasping decoding
By understanding and tuning these parameters, you possibly can optimize textual content era for various purposes, from factual writing to inventive storytelling, code era, and dialogue programs.
Source link